What Is ASP.NET MVC?
The Microsoft ASP.NET MVC framework is Microsoft’s newest framework for building
web applications. The ASP.NET MVC framework was designed from the ground up to
make it easier to build good software in the sense of good software discussed
in this chapter.
The ASP.NET MVC framework was created to support pattern-based software
development. In other words, the framework was designed to make it easier to
implement software design principles and patterns when building web
applications.
Furthermore, the ASP.NET MVC framework was designed to its core to support unit
tests. Web applications written with the ASP.NET MVC framework are highly
testable.
Because ASP.NET MVC applications are highly testable, this makes the ASP.NET MVC
framework a great framework to use when practicing test-driven development.
ASP.NET MVC Is Part of the ASP.NET Framework
Microsoft’s framework for building software applications—any type of application
including desktop, web, and console applications—is called the .NET framework.
The .NET framework consists of a vast set of classes, tens of thousands of
classes, which you can use when building any type of software application. For
example, the .NET framework includes classes for working with the file system,
accessing a database, using regular expressions, and generating images.
The ASP.NET framework is one part of the .NET framework. The ASP.NET framework
is Microsoft’s framework for building web applications. It contains a set of
classes that were created specifically to support building web applications.
For example, the ASP.NET framework includes classes for implementing web page
caching, authentication, and authorization.
Microsoft has two frameworks for building web applications built on top of the
ASP.NET framework: ASP.NET Web Forms and ASP.NET MVC (see Figure 1.1).
ASP.NET MVC is an alternative to, but not a replacement for, ASP.NET Web Forms.
Some developers find the style of programming represented by ASP.NET Web Forms
more compelling, and some developers find ASP.NET MVC more compelling.
Microsoft continues to make heavy investments in both technologies.
The Origins of MVC
The ASP.NET MVC framework is new; however, the MVC software design pattern
itself has a long history. The MVC pattern was invented by Trygve Reenskaug
while he was a visiting scientist at the Smalltalk group at the famed Xerox
Palo Alto Research Center. He wrote his first paper on MVC in 1978. He
originally called it the Thing Model View Editor pattern, but he quickly
changed the name of the pattern to the Model View Controller pattern.
The MVC pattern was first implemented as part of the Smalltalk-80 class library.
It was originally used as an architectural pattern for creating graphical user
interfaces (GUIs). The meaning of MVC shifted radically when the pattern was
adapted to work with web applications. In the context of web applications, the
MVC pattern is sometimes referred to as the Model 2 MVC pattern.
The MVC pattern has proven to be very successful. Today, the MVC pattern is used
by several popular web application frameworks including Ruby on Rails, Merb,
and Django. The MVC pattern is also popular in the Java world. In the Java
world, MVC is used in the Struts, Spring, and Tapestry frameworks.
The first major MVC framework for ASP.NET was the open source MonoRail project
(see CastleProject.org). There continues to be an active developer community
around this project.
The Microsoft ASP.NET MVC framework was originally created by Scott Guthrie on
an airplane trip to Austin, Texas, to speak at the first Alt.NET conference in
October 2007. (Scott Guthrie was one of the creators of ASP.NET.) Scott
Guthrie’s talk generated so much excitement that the ASP.NET MVC framework
became an official Microsoft product. ASP.NET MVC 1.0 was released in the first
part of 2009.
The Architecture of an ASP.NET MVC Application
An MVC application, a Model View Controller application, is divided into the
following three parts:
-
Model—An MVC model contains all of an application’s logic that is not contained
in a view or controller. The model includes all of an application’s validation
logic, business logic, and data access logic. The MVC model contains model
classes that model objects in the application’s domain.
-
View—An MVC view contains HTML markup and view logic.
-
Controller—An MVC controller contains control-flow logic. An MVC controller
interacts with MVC models and views to control the flow of application
execution.
Enforcing this separation of concerns among models, views, and controllers has
proven to be a useful way of structuring a web application.
First, sharply separating views from the remainder of a web application enables
you to redesign the appearance of your application without touching any of the
core logic. A web page designer (the person who wears the black beret) can
modify the views independently of the software engineers who build the business
and data access logic. People with different skills and roles can modify
different parts of the application without stepping on each other’s toes.
Furthermore, separating the views from the remainder of your application logic
enables you to easily change the view technology in the future. One fine day,
you might decide to re-implement the views in your application using
Silverlight instead of HTML. If you entangle your view logic with the rest of
your application logic, migrating to a new view technology will be difficult.
Separating controller logic from the remainder of your application logic has
also proven to be a useful pattern for building web applications. You often
need to modify the way that a user interacts with your application. You don’t
want to touch your view logic or model logic when modifying the flow of
execution of your application.
Understanding the Sample ASP.NET MVC Application
A good way to get a firmer grasp on the three logical parts of an MVC
application is to take a look at the sample application that is created
automatically when you create a new ASP.NET MVC project with Visual Studio.
Follow these steps:
-
Launch Visual Studio.
-
Select the menu option File, New Project.
-
In the New Project dialog, select your favorite programming language (C# or
VB.NET) and select the ASP.NET MVC Web Application template. Give your project
the name MyFirstMvcApp and click the OK button (see Figure 1.2).
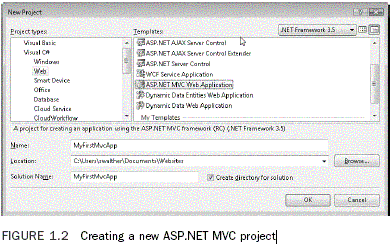
Immediately after you click the OK button to create a new ASP.NET MVC project,
you see the Create Unit Test Project dialog in Figure 1.3. Leave the default
option selected—Yes, Create a Unit Test Project—and click the OK button.
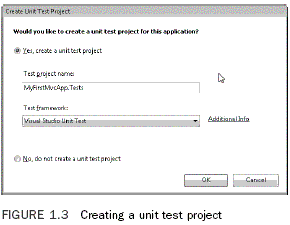
Your computer hard drive will churn for a few seconds while Visual Studio
creates the default files for a new ASP.NET MVC project. After all the files
are created, the Solution Explorer window should contain the files in Figure
1.4.
The Solution Explorer window in Figure 1.4 contains two separate projects: the
ASP.NET MVC project and the Test project. The Test project contains all the
unit tests for your application.
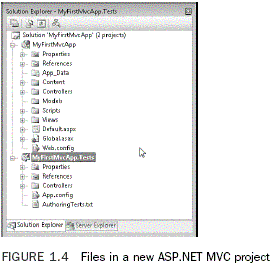
ASP.NET MVC Folder Conventions
The ASP.NET MVC framework emphasizes convention over configuration. There are
standard locations for each type of file in an ASP.NET MVC project. The ASP.NET
MVC application project contains the following folders:
-
App_Data—Contains database files. For example, the App_Data
folder might contain a local instance of a SQL Server Express database.
-
Content—Contains static content such as images and Cascading
Style Sheet files.
-
Controllers—Contains ASP.NET MVC controller classes.
-
Models—Contains ASP.NET MVC model classes.
-
Scripts—Contains JavaScript files including the ASP.NET AJAX
Library and jQuery.
-
Views—Contains ASP.NET MVC views.
When building an ASP.NET MVC application, you should place controllers only in
the Controllers folder, JavaScript scripts only in the Scripts folder, ASP.NET
MVC views only in the Views folder, and so on. By following these conventions,
your application is more easily maintained, and it can be more easily
understood by others.
Also read
First
ASP.NET MVC Application
Receive an overview of all of the aspects of an ASP.NET MVC web application.
Explore the ASP.NET MVC web application project template that is installed in
Visual Studio 2008. Create a fi rst action method and a corresponding view.
Create a strong-typed view.............. |
Model
View Controller
Understanding the Page Controller pattern
Understanding the need for the MVC design pattern
Learning the basics of MVC design
Understanding the Front Controller design pattern..............
|
Components
of MVC architecture
Model: In the MVC pattern, the component,” model” comprises the code that is to
deal with the database (can be a simple java bean). It is built without
appearance concerns at the time of presenting to the user.................
|
Benefits of MVC pattern
Separation of concerns:
- The separation the three components, allows the re-use of the business logic
across applications.............
|
|