How to draw different types of shapes using 2D-graphics in Android? Explain with example.
The Android provides a huge set of 2D-drawing APIs that allow you to create graphics.
There are two ways to create graphics using these API.- Drawing to a View
- Drawing on a Canvas
If you want to draw graphics that have static graphic content or predefined animation, then draw your graphics or animations into a View object from your layout.
If you want to draw graphics that have dynamic content such as video games, then draw your graphics directly to a Canvas. The Canvas class has several methods for drawing lines, bitmaps, text and many other different types of shapes.
Some of the important methods of canvas class are as follows.- drawText()
- drawRoundRect()
- drawCircle()
- drawRect()
- drawBitmap()
- drawARGB()
You can use these methods in onDraw() to create your custom user interface.
Following is the content of the modified MyView.java file
Create a new java class that should extend from View class. Override the
onDraw() method. In this method you can use
Canvas class to draw the different shape.
publicclassMyViewextends View
{
publicMyView(Context context)
{
super(context);
// TODO Auto-generated constructor stub
}
@Override
protectedvoidonDraw(Canvas canvas)
{
// TODO Auto-generated method stub
super.onDraw(canvas);
int radius;
radius = 50;
Paint paint = newPaint();
paint.setStyle(Paint.Style.FILL);
paint.setColor(Color.parseColor("#CD5C5C"));
canvas.drawCircle(150,200, radius, paint);
canvas.drawRoundRect(newRectF(20,20,100,100), 20, 20, paint);
canvas.rotate(-45);
canvas.drawText("CareerRide", 40, 180, paint);
canvas.restore();
}
}
Following is the content of the modified MainActivity.java file
Note that you have to pass the object of sub class that extends from View class in
setContentView() method as given below. In our case the name of sub class is MyView.
publicclassMainActivityextends Activity
{
@Override
protectedvoidonCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(newMyView(this));
}
@Override
publicbooleanonCreateOptionsMenu(Menu menu)
{
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
returntrue;
}
}
Execute the above code, you will the output as given below.
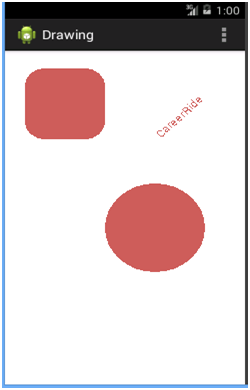