Java Multithreading for Computer Science and MCA students
Which methods are used for interthread communication?
- The interthread communication is a technique through which one thread produces some data and another thread produces some data and another thread is ready to consume it.
- It provides an efficient way through which multiple threads communicate with each other by reducing the CPU idle time.
- It implements the various methods that are defined in the java.lang package and can be called within the synchronized code only.
Following are the methods used by interthread communication:- wait() : Here the calling thread is commanded to discard the monitor and go to sleep until some other thread enters the same monitor and calls the notify().
- notify() : It wakes up the the first thread that called the wait() on the same object.
- notifyAll() : It wakes up all the threads that called the wait() on the same object. The thread with the highest priority runs first.
All these methods can be called only from within a synchronized context. They are declared within the Object class.
An
example for this can be the Producer,Consumer and Stock. The Stock will contain the buffer stock of the goods. The goods produced by the producer are received and added in the buffer stock. The consumer takes the goods from the buffer stock. The stock acts as a central class to add the goods and takes away the goods from the buffer stock.
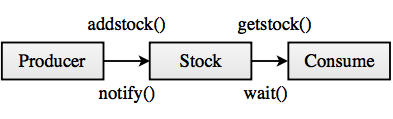
Write a note on Thread life cycle.
- Like life cycle of a creature on the earth, threads also have life cycle. In that life cycle thread goes through different states viz.
- Threads follow the single flow of control. A thread is execution in a program.
The life cycles of threads are listed below:- Newborn state: After the creations of Thread instance the thread is in this state but before the start() method invocation. Thread is considered not alive in this phase.
- Runnable state: A thread starts its life from Runnable state. After the invoking of start() method thread enters Runnable state.
- Running state: A thread first enters Runnable state.
- Blocked state: A thread can enter in this state because of waiting the resources that are hold by another thread.
- Dead state: A thread can be considered dead when its run() method completes. If any thread comes on this state that means it cannot ever run again.
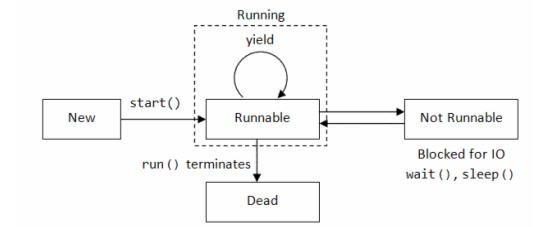