What is OOP? What are the key features of OOP?
- OOP is commonly known as Object Oriented Programming.
- It is a programming technique that is associated with the concept of Class, Object and various other concept revolving around the concepts like Inheritance, Polymorphism, Abstraction, Encapsulation etc.
Key features of OOP are:- Emphasis is on data rather than procedure.
- Programs are divided into entities as objects.
- Data structures are designed in such a manner that the objects are characterized.
- Function that operate on data of an object are tied together in data structures.
- Data is hidden and cannot be accessed by external functions.
- Objects communicate with each other through functions.
- If it's necessary to add new data and functions, then they can be added easily.
- In program design, the bottom up design approach is followed.
What are the advantages of OOP?
- OOP supports the modular structure for developing applications.
- To hide the inner details good definitions, abstract data types are implemented.
- Modifying the existing code is easier. The existing code can be modified with minimal differences to existing objects.
- It provides a good framework of code library where software components can easily be adapted and modified.
What are the basic concept used in the Object Oriented programming language?
Class- A class represents description of objects that share same attributes and actions.
- It defines the characteristics of the object, such as attributes, actions or behaviors.
- It is the blue print of an object.
Object- An object is an instance of a class.
- An object is an entity that keeps together state and behaviors.
- All the instance of a class have similar properties like class definition.
For example:
Create instances of the class student for s1,s2,s3.
Data Abstraction- An abstraction is a representation of essential features by hiding the background process or details.
Encapsulation- Encapsulation means hiding the data of a class from the object.
- It is the process of binding the elements of an abstraction.
- It binds the data in a single unit.
Polymorphism- The ability to exist in various forms is called as polymorphism or Polymorphism means the ability to take more than one form.
Inheritance- Inheritance is a technique of deriving a new classes from existing one.
- It increases code reusability.
Message passing- Sending and receiving information among objects through function parameters is known as Message Passing.
- Object communicates through invoking methods and sending data to them.
Dynamic binding- C++ provides facility to specify that the compiler should match function calls with the correct definition at the run time it is called dynamic binding.
- It also refers to as late binding.
What is a ternary operator?
- The '?' is called as ternary operator because it requires three operands.
- It is used instead of if-else statement.
- It is also called as a conditional operator.
Syntax:
(expression1) ? (expression2) : (expression3);
This means expression 1 is true then return the value of expression 2 otherwise returns the value of expression 3.
Example:
#include<iostream>
using namespace std;
void main()
{
int z;
z = (5> 8) ? 30:60;
cout<<”Result is: “<<z;
}
Output:
Result is: 60
What is an abstraction and why is it important?
- An abstraction is a representation of essential features by hiding the background process or details.
- Abstraction is important at the conceptual level of an application.
- It helps to clearly understand the process and the flow of an application.
- The abstraction allows the complete flow and development of an application into a structured action.
- The conceptual level of project execution is derived from abstraction which is the gateway for the entire application development.
What are the examples of tokens?
Keywords
These are some reserved words in C++ which have predefined meaning to compiler called keywords.
Identifiers- A symbolic name is known as an identifier.
- The identifier is a sequence of characters taken from C++ character set.
The rule for the formation of an identifier are:- An identifier can consist of alphabets, digits and/or underscores.
- It must not start with a digit.
- C++ is case sensitive that is upper case and lower case letters are considered different from each other.
- It should not be a reserved word.
Constants- Integer-Constants
- Character-constants
- Floating-constants
- Strings-constants
Operators
Operators are special symbols used for specific purposes.
C++ provides six types of operators- Arithmetical operators
- Relational operators
- Logical operators
- Unary operators
- Assignment operators
- Conditional operators
- Comma operator
Punctuators
It includes
- Brackets [ ]
- Parentheses ( )
- Braces { }
- Comma ( , )
- Semicolon ( ; )
- Colon ( : )
- Asterisk ( * )
- Equal sign ( = )
- Pound sign (#)
What is friend function?
- If a function is friend of a class, then it allows to access Public, private or protected data of that class is known as friend function.
- It can be declared anywhere in the class declaration.
- It doesn’t have any effect on access of the control keywords like private, public or protected.
Syntax:
friend returntype functionname(datatype of argument);
What are the different ways for a method to be overloaded?
A method can be overloaded by:- Varying the numbers of parameters.
- Using different data types for the parameters.
- Using different sequence of the parameters.
What is the function of dynamic typing?
- Dynamic typing is a way to allow the compiler to replace an existing value with a type that is not functioning properly.
- There can be warning signs like: Incompatible types in assignment.
- This warning sign shows that there is type checking that casts the object from one to another to make it compatible with the current system.
- The dynamic typing checks for the type of object and during the compile time and the run time the value doesn't change.
- If the class of the variable can be found during the run time then it will be possible to find out the class of the variable.
- Dynamic binding provides the output.
- It allows the binding between the objects to be done at runtime.
What is Information Hiding in OOP?
- Information hiding concept restricts direct exposure of data.
- Data is accessed indirectly using safe mechanism, methods in case of programming object.
- Information hiding isolates the end users from the requirement to intimate the knowledge of design for the use of a module.
What are the types of access specifier?
Public- If a class member is public, then it can be used in the class as well as outside the class without access restrictions.
- Generally member functions are kept public.
Private- If a class member is private, then it can be accessed only within the class that is only by the members and friends of class.
- Generally data members are kept private because programmers wants to hide internal details of object.
Protected- If a class member is protected, then it can be accessed in the class as well as in all the derived classes which are from that class.
Explain the desirable qualities of an object.
Modularity- The operations or methods should be defined separately for each module of the software project.
- One of the objects feature can be used by other object as a reusable component.
Information Hiding- The data about one object should exclusively belong to that object, which enables the individual module to focus on specific functionality.
Re-usability of code- An object of one class should have the same functionality that of another.
- The code is re-usable by all objects of one class.
- There should not be mismatching of the functionality of one object to that of another.
What is function overloading?
- Defining multiple function in a program having same name is called as function overloading.
- Function must have different number or type of argument.
- The function to be called depends upon the number of arguments passed while calling the function.
For example:
#include<iostream.h>
int volume(int l,int b,int h) // volume of box
{
int v = l * b * h;
return v;
}
int volume(int n) // volume of cube
{
int v = n * n * n;
return v;
}
void main()
{
int a = volume(5,7,8);
cout<<”volume of box is ”<<a;
int b = volume(5);
cout<<”volume of cube is “<<b;
}
In the above example, volume function created twice with different number of arguments that are passed to the function and the compiler decides according to the arguments that are passed through the function which function is to be called.
What is the syntax of object oriented method call?
- The object oriented syntax tells about the object oriented features and methodologies that are present and shown as:
target.method(arguments);- This is the line that can be used to call the functions, but not invoking the methods on an object.
- A function is made up of both the operands and operators and they are very essential to call a method. So, the method will be written as:
a = sub(c,b);- Object oriented syntax allows easy to write ways and some specified rules that has to be remembered while programming.
- The operator has to always come in the middle of the statement.
- A class is made for every module and it also represents the template used for a class made up of objects.
What is a constructor?
- Constructor is a special member function of a class that executes automatically whenever an object is created.
- It is a member of the class and has same name as the class name.
- An object’s constructor is automatically invoked whenever the object is created.
- Constructor don't have any return type not even void.
- It allows initialization of objects at the time of constructor creation.
- Constructors are always public. They are used to make initializations at the time of object creation.
Explain destructor.
- A destructor is a special member function of a class.
- It is executed when an object of the class goes out of scope.
- Destructor are usually used to deallocate memory and releasing resources before coming out of the program.
- A destructor is called for a class object when that object passes out of scope or is explicitly deleted.
- A destructor takes no arguments and has no return type.
- A destructor has a same name as the class prefixed with a tilde (~) and it doesn't take any argument or not return any value.
What are the types of constructors?
There are three types of constructors:
1. Default constructor
2. Copy constructor
3. Parameterized constructor
1. Default constructor:- It has no argument and it is automatically called when creates the object of a class.
- It is also called as empty constructor.
- It is called during default initialization.
2. Copy constructor:- It is used to initialize an object by using data of another object of same type.
- The copy constructor is automatically called when an object is assigned to it.
- The reference of object which is assigned and it comes as an argument to copy constructor.
- Copy constructor allows creating new objects from existing objects by initialization.
3. Parameterized constructor:- In parameterized constructor,the data is passed while creating an object.
- This data is passed to the constructor as an argument and it is used for initialization of the object.
- If the class contains a constructor with argument and it do not have any default argument then it is compulsorily to call parameterized constructor by passing data.
What is inline function?
- An inline function is a combination of macro and function.
- At the time of declaration or definition, function name is preceded by word inline.
- When inline functions are used, the overhead of function call is eliminated.
- Instead, the executable statements of the function are copied at the place of each function call. This is done by the compiler.
What is inheritance? What are the types of inheritance?
- Inheritance is technique of deriving a new classes from existing one.
- The derived class inherits all the features of base class from which it is declared.
Types of Inheritance:
1) Single Inheritance
2) Multiple Inheritance
3) Hierarchical Inheritance
4) Multilevel Inheritance
5) Hybrid Inheritance
What is function overriding?
- Redefining a base class function in derived class is called function overriding.
- The overrided function must have same name, same number and type of argument.
- This overrided function is called for derived class object instead of the inherited class function.
- The function marked public, final or static can not be overridden.
What is composition or containership?
- The objects of existing class inside the new class is called composition.
- The new class is composed of object in the existing classes so it is known as composition.
- The data member of a new class is an object of some other class.
Explain types of inheritance.
1. Single level inheritance:- If a class is derived from a single class then such type of inheritance is called single level inheritance.
- It is the process which allows the objects of one class to acquire the properties of objects in another class.
- The class that inherits is called sub-class or derived while the class from which the object is inherited is called superclass or base class.
2. Multi level inheritance:- If the class is derived from a derived class then such type of inheritance is called as single level inheritance.
3. Hierarchical inheritance:- If the multiple classes are derived from single class then such type of inheritance is called as hierarchical inheritance.
4. Multiple inheritance:- If a single class is derived from multiple classes then such type of inheritance is called as multiple inheritance.
5. Hybrid inheritance:- If mixed type of inheritance is used then it is called as hybrid inheritance.
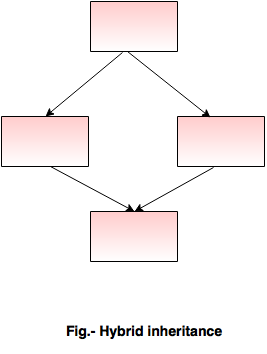
What are the advantages of inheritance?
- It Allows the code to be reused as many times as needed.
- The base class once defined and compiled then it need not be reworked.
- It saves time and effort as the main code need not be written again.
Explain dynamic Memory Management.
C++ provides two new operator 'new' and 'delete' to perform dynamic memory management.
New:- This operator reserves a block of memory at runtime.
- It reserves a block of memory required for specified datatypes and returns the starting address.
Syntax:
pointer = new datatype;
For example:
1) int *p;
p = new int;
It dynamically reserve 2 bytes & store its address into p.
2) char *x;
x = new char[50];
It dynamically reserve 50 bytes and store its address into x.
3) student *z;
z = new stud(3117, 'ram');
It dynamically create a object and store it into p.
delete:
This operator is used to release memory which was dynamically reserved by the new operator.
syntax:
delete pointer;
It released memory block of specified pointer.
Explain 'this' pointer.
- In c++, every object accesses its own address through an important pointer is called as 'this pointer'.
- This pointer is used as a self reference within a class.
- This pointer is used when user want address of the object itself within the class.
- It is generally used to access its own member if names conflict, to return an address of object or object itself.
What is polymorphism and how it can be achieved?
- Polymorphism is the ability of different classes to expose similar interfaces to the outside.
- It means that single statement can have different meaning at different time.
- Polymorphism can be achieved through dynamic linking or late binding.
- To achieve polymorphism through late binding C++ provides a features of a base class pointer can point to object of its derived class. Using that pointer can access only those member which are declared in base class.
What is static linking and dynamic linking?
Static linking:- In static linking, the function to be called is decided at compile time depending on type of pointer and not object.
- It is also called as early binding.
- In C++, early binding is performed by default.
Dynamic linking:- In dynamic linking, the function to be called is decided at run time depending on the type of object and not pointer.
- It is also called as late binding.
Why dynamic binding is used in object-oriented programming?
- Dynamic binding allows delaying the decision of running the program.
- Dynamic binding is used to perform messaging transparently and routinely by allowing different objects to be binded on the run time.
- It allows the declared pointer to find out the assigned values of a give procedure.
- Messages that are given with the programs are invoked indirectly.
- Every method has a message expression that has to be found for implementation.
- The class of the receiver is checked to locate the implementation of the method that is mentioned in the message.
- The complier dynamically bind the objects at run time the method.
What is the function of messaging metaphor?
- Object oriented programming is having its own metaphors that are associated with the program or the code that has a role to play in the program.
- Messaging metaphor represent the relationship between the actors that are involved in it and the roles played by each.
- This allows easy passing of the responsibilities and information to the processes that are in contact with the one which is passing the information.
- Messaging method allows the user to use the objects rather than their functions.
- The objects perform all the actions that is been done by the methods or the functions alone.
- Objects consists of state and behavior so they are not active rather passive, but they act as an agent to allow the program's activity to be performed easily.
- The metaphor keeps all the objects separate each with the defined roles.
What is the Aggregation and Composition?
Aggregation:- Aggregation is a collection of the entire program.
- It consists of only one object and this object sends messages to itself and other objects by using the method of polymorphism.
- The modularity concept is used for the classes and program design to set the network of objects that are interconnected to each other.
- In Aggregation, the program is hidden from the class definition.
Composition:- Composition is a way to combine all the small sub-components to provide the functionalities in modular format.
- This makes the structure less usable.
Why message passing between the objects is important?
- Objects use the metaphor concept to pass the messages between different objects.
- The objects communicate through the messages only. The first step is to call the method as the functions are called in a normal way.
- The moment a function is called by an object a message will be passed to all the objects to allow the operations to be performed.
- Then the abstract method is separated from the particular data to show the correct behavior of the state. Object oriented programming keeps a start function that initiates the operation.
- Then the information that is contained in the object is archiving and then the program gets executed according to the method or the function. The different objects will have different functions to be performed.
- There are few abstract behaviors and to make it concrete the method has to be associated with an object.