ASP.NET MVC Framework
Sample Project
URL Routing Engine
ASP.NET MVC Framework
The ASP.NET MVC framework was released by Microsoft as an alternative approach
to web forms when creating ASP.NET based web applications. The ASP.NET MVC
framework is not a replacement or upgrade of web forms, but merely another way
of programming your web applications so that we can get the benefi ts of an MVC
design with much less effort.
As of now, the ASP.NET MVC framework is still in CTP (Community Technology
Preview, which is similar to an advanced pre-stage), and there is no certain
date when it will be released. But even with the CTP 5, we can see how it will
help MVC applications follow a stricter architecture.
We will quickly see how to use the ASP.NET MVC framework through a small
example.
Sample Project
First, download the ASP.NET MVC framework from the Microsoft website and install
it. This installation will create an MVC project template in VS 2008.
Start VS 2008, select the File | New Project menu item and then choose the
ASP.NET MVC Web Application template to create a new web application using this
template.
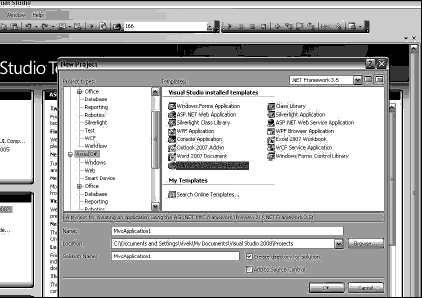
By default, when you create a new application using this option, Visual Studio
will ask you if you want to create a Unit Test project for your solution, with
a drop-down list pre-populated with the different possible types of test
frameworks (the default would be VS test, but in case you installed MBUnit or
NUnit, these would also be populated here):
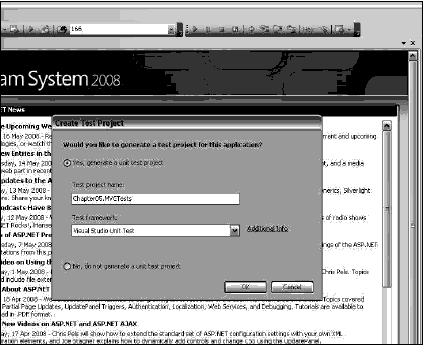
Select the default option and click OK. You will notice that two projects have
been added to the solution that VS has created. The fi rst project is a web
project where you'll implement your application. The second is a testing
project that you can use to write unit tests against.
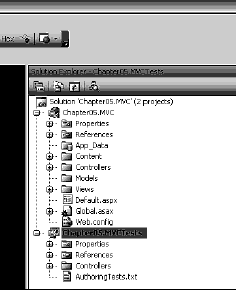
In our custom MVC code project, we had different projects (class libraries) for
the model, the view, and the controllers.
The default directory structure of an ASP.NET MVC Application has three
top-level directories:
-
/Controllers
-
/Models
-
/Views
When the project becomes large, it is recommended that the Model, Views and
Controllers are put in separate class library projects of their own so that
it's easy to maintain them. But for the purpose of illustrating the ASP.NET MVC
framework, this default structure is fi ne for us.
We will create a simple customer management application. For this, we fi rst
create some ASPX pages in the Views folder. Note that VS has already created
these subfolders for us, under Views:
-
Home: Contains the and Index views
-
Shared: Contains shared views such as master pages
Before we go on to adding custom code in this project, let us understand what VS
has done for us while creating this MVC project.
URL Routing Engine
In the standard ASP.NET model (or Postback model), the URLs map directly to the
physical fi les:
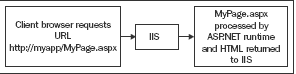
So when we make a request to a page, say MyPage.aspx, the runtime compiles that
page and returns the generated HTML back to IIS to be displayed by the client
browser. So we have a one-to-one relationship between the application URLs and
the page.
But in the MVC framework, the URLs map to the controller classes.
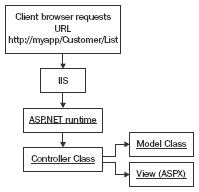
Therefore, the URL is sent to IIS and then to ASP.NET runtime, where it
initiates a controller class based on the URL, using the URL routes, and the
controller class then loads the data from the model, with this data fi nally
being rendered in the view.
The controller classes uses URL routing to map the URLs, which in simpler terms
means rewriting URL. We can set up the rules for which URL is to be routed to
which controller class. The routing will pick up the appropriate controller and
pass in the query string variables as necessary.
Open the global.asax.cs file and examine the following code:
public class GlobalApplication : System.Web.HttpApplication
{
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
"Default",
// Route name
"{controller}/{action}/{id}",
// URL with parameters
new
{ controller = "Home", action = "Index", id = ""
}
//
Parameter defaults
);
}
protected void Application_Start()
{
RegisterRoutes(RouteTable.Routes);
}
The RegisterRoutes() method contains the URL mapping routes. Initially we have
only the default rule set:
routes.MapRoute(
"Default",
// Route name
"{controller}/{action}/{id}",
// URL with parameters
new
{ controller = "Home", action = "Index", id = "" }
// Parameter
defaults
);
This URL mapping engine comes from System.Web.Routing.dll, which can be used
independently, without the ASP.NET MVC framework, to rewrite URLs in your
standard ASP.NET web applications.
The MapRoute() method, which handles URL routing and mapping, takes three
arguments:
-
Name of the route (string)
-
URL format (string)
-
Default settings (object type)
In our case, we named the fi rst route "Default" (which is the route name) and
then set the URL as:
Controller/action/id
The Controller here is the name of the controller class. action will be the
method that needs to be invoked inside that controller class. id would be the
parameters that need to be passed, if any.
In the default arguments, we create a new object and call it "Home", set the
action to Index, and do not pass parameters to it. Note the new anonymous type
syntax used to create parameter defaults:
new { controller = "Home", action = "Index", id = "" }
It is important that we name and assign a value to each of the properties that
we are creating. What will be the type of the properties? They will
automatically be cast to the data types of the values of the properties specifi
ed. The anonymous types will always be derived from the base object class
directly. They can only be used within class members and cannot be passed as
method arguments (unless they are boxed), return values, or be specifi ed as
class-level variables. Once the type is created, it cannot be changed into
another type.
So we create a new anonymous type as the last argument of the MapRoute() method,
passing in variable defaults with three properties, namely controller, action,
and parameter.
Now have the Default.aspx page under the root directory, which acts as a
redirecting page to the main home page of the site (which is
/View/Home/Index.aspx).
We cannot directly set that as the "default" page since we are using URL routes
to process pages instead of using physical fi les in the URLs.
So in the code-behind of our Default.aspx page, we have a simple redirect:
public void Page_Load(object sender, System.EventArgs e)
{
Response.Redirect("~/Home");
}
So the runtime will fi rst set up routes in the global.asax page, then it will
process the Default.aspx page. Here it faces a redirect to this URL: /Home.
The Controller
The MVC framework maps this URL to the route set in the global route table,
which currently has only the default one, in this format:
Controller/action/id
So /Home corresponds to a controller named Home, and because we have not specifi
ed any action or ID, it takes the default values we specifi ed in the
RegisterRoutes() method in the globals.asax.cs. So the default action was Index
and the default parameter was an empty string. The runtime initializes the
HomeController.cs class, and fi res the Index action there:
public class HomeController : Controller
{
public ActionResult
Index()
{
ViewData["Title"] = "Home Page";
ViewData["Message"] = "Welcome to ASP.NET MVC!";
return View();
}}
In this Index() method, we set the data to be displayed in the View (aspx/ascx
pages) by using a dictionary property of the base Controller class named
ViewData. ViewData, as the name suggests, is used to set view-specifi c data in
a dictionary object that can hold multiple name/value pairs. When we call the
View() method, the ViewData is passed by the Controller to the View and
rendered there.
The View
Let us now look at the View. How does the framework know which View or aspx page
to call? Remember that we passed the value "Index" in the action parameter (in
the default route in the global.asax.cs fi le), so the Index.aspx will get
called. Here is the code-behind of Index.apsx:
public partial class Index : ViewPage
{
}
There is absolutely no code here, which is a very important characteristic of
the MVC design. The GUI should have no logical or data fetching code. Note that
the Index class is derived from the ViewPage class. Using this ViewPage class,
we can access all of the items in the ViewData dictionary that were set in the
controller's Index() method and passed on to the View. Here is how we are
accessing the ViewData in HTML:
<asp:Content ID="indexContent" ContentPlaceHolderID="MainContent"
runat="server">
<h2><%= Html.Encode(ViewData["Message"]) %></h2>
<p>
To learn more about ASP.NET MVC visit <a href="http://asp.net/mvc"
title="ASP.NET MVC Website">http://asp.net/mvc</a>.
</p>
</asp:Content>
We can directly access the ViewData dictionary in HTML. Now that we have seen
how MVC works, we will create a new page to learn how to show data using a
custom DAL and strongly typed objects, instead of the ViewData dictionary. Our
example page will show a list of all the customers.
The Model
We will use the 5-Tier solution we created in the previous chapter and change
the GUI layer to make it follow the MVC design using the ASP.NET MVC framework.
Open the solution we created in the previous chapter and delete the ASP.NET web
project from it. The solution will then only contain 5Tier.BL,
5Tier.DAL and 5Tier.Common projects.
Right click the solution in VS, and select Add New Project, and then select
ASP.NET MVC Web Application from the dialog box. Name this new web project as
Chapter05.MVC. This web project will be the new MVC based UI tier of our OMS
application in this chapter.
The Customer.cs and CustomerCollection.cs class fi les in the business tier
(5Tier. Business class library) will be the Model in our MVC application. To
show a list of customers, the CustomerCollection class simply calls the
FindCustomer() method in CustomerDAL.cs. We have already seen these classes in
action in the previous chapter. So we can use an n-tier architecture in an MVC
application, hence this shows that MVC and n-tier are not mutually exclusive
options while considering the application architecture of your web application.
Both actually compliment each other.
We can also create a utility class named CustomerViewData to transfer the Model
objects to the View. There are multiple ways to pass- in the Model to the View
through the Controller, and creating ViewData classes is one of them. Here is
the CustomerViewData class created in the CustomerComtroller.cs fi le in the
Chapter05.MVC web project:
#region ViewData
/// <summary>
/// Class used for transferring data to the View
/// </summary>
public class CustomerViewData
{
public
CustomerViewData() { }
public
CustomerViewData(Collection
customers)
{
this.customers =
customers;
}
public Collection
customers;
public Customer customer; } #endregion
Notice that this ViewData class is simply wrapping the business object inside it
so that we can use this class in the UI layer instead of directly passing and
manipulating domain objects.
<<Previous
More Related links
What Is ASP.NET MVC?
The ASP.NET MVC framework was created to support pattern-based software
development. In other words, the framework was designed to make it easier to
implement software design principles and patterns when building web
applications..............
Your First ASP.NET MVC Application
This chapter describes how to use this template. We will briefl y touch all
aspects of ASP.NET MVC by creating a new ASP.NET MVC web application based on
this Visual Studio template. Besides view, controller, and model, new concepts
including ViewData—a means of transferring data between controller and view,
routing—the link between a web browser URL and a specifi c action method inside
a controller, and unit testing of a controller are also illustrated in this
chapter.
ASP.NET Session
State Management questions with answers
Define Session, SessionId and Session State in ASP.NET. |
What is Session Identifier? | Advantages and disadvantages of using Session
State Management. | What are the Session State Modes? | Define each Session
State mode supported by ASP.NET.
ASP.NET Validation
Control questions with answers
Define Validation Control in ASP.NET. | What are the
validation types supported by ASP.NET? | Describe the steps to use Validation
Control.
Interview
questions and answers on .Net web service
What is XML Web Server? | Describe the Components that help
locate and consume XML Web Service. | Describe the process of communication
between Client and Web Service. | What are the components published while
deploying of Web Service? | Describe .disco file. | Describe the step to be
followed to access web service by client.
ASP.NET 2.0 Security Controls
With ASP.NET 2.0, things have changed for the better. For security-related
functionalities, ASP.NET 2.0 introduces a wide range of new
controls..............
ASP.NET 2.0 New Controls
In addition to the new controls, ASP.NET 2.0 also provides numerous enhancements
to existing controls that make these controls more versatile than ever before
in building component-based web pages. For example, the Panel control now has a
DefaultButton property that specifies which button should be clicked if the
user presses the Enter key while the panel has the focus.........
ASP.NET 2.0 Validation Groups
With ASP.NET 2.0, Microsoft introduces a new feature known as validation groups,
which enables you to create different groups of validation controls and assign
them to input controls, such as text boxes. You can assign a validation group
to a collection of input controls if you want to validate the collection of
input controls on the same criteria............
|