Advance Java Questions for Computer Science Students
What is the difference between JDBC and ODBC?
JDBC | ODBC |
---|
JDBC stands for java database connectivity. | ODBC stands for open database connectivity. |
It is used for java applications. | It is used for database. |
JDBC is directly used with java. | ODBC can't directly used with java because it has C interface. |
JDBC doesn't support pointers. | ODBC supports pointers. |
JDBC driver are in built and come with the java installation. | ODBC drivers has to be installed manually on all the client machines. |
Advantages and disadvantages of Java Sockets.
Advantages of Java Sockets :- Sockets are flexible and easy to implemented for general communications.
- Sockets cause low network traffic.
Disadvantages of Java Sockets :- Socket based communication allows only to send packets of raw data between applications.
- Both the client-side and server-side have to provide mechanisms to make the data useful in any way.
What is the difference between multiple processes and multiple threads?
Multiple processes | Multiple threads |
---|
Multiple processes provide multitasking environment and allows the user to switch different program quickly. | Multiple threads share the same variable and same data. |
Multiple processes are safe to use. | Multiple threads are riskier because they share the same data. |
Multiple processes have more overhead. | Multiple threads have less overhead. |
In multiple processes, inter communication is slower and more restrictive | In multiple threads communication between threads are faster. |
On which platform J2EE is based?
- J2EE stands for Java 2 Enterprise Edition.
- It provides a component based approach to design, development, assembly and deployment of enterprise applications.
- J2EE provides advanced features to be used by the user.
- It provides multithreading and multi-tired environment to support distributed application model.
- It also defines the unified security model and provides the support for integration of XML (Extensible Markup language), which is based on open standards and protocols.
What is the purpose of JSP and why it is widely used?
- JSP stands for Java Server pages.
- It is used to create dynamic web pages.
- JSP is one of the most widely used language for the creation of web pages.
- It allows the dynamic component to be added and it includes servlets that are used to execute dynamic codes.
- It is used to create static as well as dynamic content.
- It provides high level.
How servlets are deployed in Java?
- Servlets are Java classes that are used to store codes and create applications to be used by JSP for execution.
- These programs run on a web server and they are used to act as a middle layer.
- It takes the request and pass it to the server and gives the reply to the HTTP client.
- It is used to extend the capability of servers.
- They are used with HTTP protocol and to define the client server architecture.
- It is used to add the dynamic content to a web server using the java run time libraries and java platform.
What is the purpose of a web-client?
- The main purpose of the web-client is to create dynamic web pages.
- It consists of various types of markups like HTML, XML, and PHTML.
- It also generates the web components that are running on the web server.
- It allows the web browser to read and render the pages that are received from the server.
- It helps easy communication between the client and other clients that are connected to each other.
What are the jobs performed by servlets?
- Servlets are used for the web servers to receive and reply to the request that is sent by the user.
- The request comes from the HTTP client and the servlet code only delivers it to the HTTP server and brings back the reply and passes it to the client.
- The servlet includes the information and details about the browser, cookies, host name and other resources.
- It is also used to generate the results by communicating with the database.
- It allows the formatting to be done inside a document.
- It sets the HTTP response parameter so that the browser will get to know what type of document is being returned.
- It returns the reply to the client in the HTML format.
What are the advantages of servlets over traditional CGI?
The advantages of servlets over traditional CGI are as follows:- Servlets are a more efficient way to represent the data.
- CGI(Common Gateway Interface) creates an overhead on the process and execution time can be hindered.
- Java Virtual Machine uses a lightweight java thread to handle the request, whereas CGI uses heavy weight operating system process.
- The data loaded in the memory in the case of CGI program is heavier than the servlets.
- CGI program terminates after handling the request, whereas servlet remains in the memory and store the complex data between the requests.
- Servlets are more convenient way to use the infrastructure and allow automatic parsing and decoding of HTML form data but it is not the case with CGI.
- Servlets are more portable and secure and follow the standard API but CGI programs are executed by general purpose shells that are not very much secure.
What is the purpose of apache tomcat?
- Apache server is a standalone server that is used to test servlets and create JSP pages.
- It is free and open source that is integrated in the Apache web server.
- It is fast, reliable server to configure the applications but it is hard to install.
- It is a servlet container that includes tools to configure and manage the server to run the applications.
- It can also be configured by editing XML configuration files.
What is the purpose of HttpClient?
- HttpClient is a class that is used for network communication by sending required request line and headers to the web server.
- It places the request lines in a text area until the communication get lost or the connection get closed.
- The connection can be closed if HttpClient gets interrupted by isInterrupted flag.
How the interruptible method gets implemented?
- Interruptible is an interface that is used to identify certain classes, which have an isInterrupted method.
- It is used by HttpClient to see the interruption done by any user or for the webclient.
- Its syntax is given as:
public interface Interruptible
{
public boolean isInterrupted();
}
Where is pragma used?
- Pragma is used inside the servlets in the header with a certain value.
- The value is of no-cache that tells that a servlets is acting as a proxy and it has to forward request.
- Pragma directives allow the compiler to use the machine and operating system features while keeping the overall functionality with the Java language. These are different for different compilers.
What are different core interfaces of Hibernate Framework?
Hibernate applications interact with the applications that are being provided by Hibernate core:- org.hibernate.Session: This manages the operations of storing and retrieving the objects. It acts as a persistent manager. It obtains its session from the session factory.
- org.hibernate.SessionFactory: It is a heavy weight instance, that creates one instance for the overall application. If there are multiple databases, then one instance is required per database.
- org.hibernate.Criteria: It provides a way to do the search which is conditional over the resultset. Session factory contains criteria instances.
- org.hibernate.Query: It is the representation of Hibernate query which is object oriented. It is being created by calling Session.createQuery().
Why a dead thread occurs?
Dead thread occurs due to two reasons:
1. A thread becomes dead when the function exits normally after execution.
2. A thread becomes dead due to some exception and then terminates itself.
- A thread can be dead by calling the kill or stop method.
- The method which is being called throws a thread-death error object which sends the message for the kill.
- A thread is dead or not use, then isAlive method which returns true if the thread is runnable or blocked, in other cases it shows false.
On which platform J2EE is based?
- J2EE stands for Java 2 Enterprise Edition.
- It provides a component based approach to design, development, assembly and deployment of enterprise applications.
- J2EE provides advanced features to be used by the user and it provides multithreading and multi-tired environment to support distributed application model.
- It also defines the unified security model and provides the support for integration of XML (Extensible Markup language), which is based on open standards and protocols.
What is JCA in java?
- Java Cryptography Architecture term from Sun for implementing security functions for the Java platform.
- It provides a platform and gives architecture and APIs for encryption and decryption.
- JCA is used by the developer to combine the application with the security measure. A programmer uses the JCA to meet the security measure.
- It helps in performing the third partly security rules.
- It uses the hash table, encryption message digest, etc. to implement the security.
What is JSP?
- JavaServerPages is server side component in a web application, used to dynamically generate HTML / XML documents.
- JSP allows the java code dynamically embedded within a web page like HTML and gets executed when the page is served by the server.
- After the page is served, the dynamic content is sent to the client.
- With the script, the page is run on the server before it reaches to the client.
- JSP are used to invoke the built-in functionalities.
- In addition to the HTML, the JSP technology adds a tag library which provides a platform independent extending capabilities of a web server.
Explain the life-cycle methods of JSP?
The life-cycle methods of the JSP are detailed below :
jspInit() :- The container calls the jspInit() for initializing the servlet instance.
- It is called before any other method, and is called only once for a servlet instance.
jspService() :- The container calls the _jspservice() for each request and passes the request and the response objects.
- jspService() method can not be overridden.
jspDestroy() :- The container calls jspDestroy() when its instance is about to be destroyed.
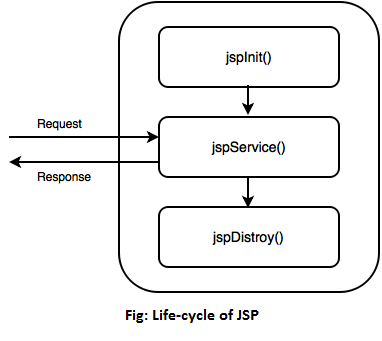
What are the implicit Objects available?
- Implicit objects are also called as pre-defined variable.
- Implicit objects are valid for only JSP pages.
- The web container creates it.
- It comprises the information related to a specific request, page & application.
The JSP implicit objects are listed below :
1. application(javax.servlet.ServletContext) : The context for the JSP page's servlet and any Web components contained in the same application.
2. config(javax.servlet.ServletConfig) : Initialization information for the JSP page's servlet.
3. exception(java.lang.Throwable) : Accessible only from an error page.
4. out(javax.servlet.jsp.JspWriter) : The output stream.
5. page(java.lang.Object) : The instance of the JSP page's servlet processing the current request. Not typically used by JSP page authors.
6. pageContext(javax.servlet.jsp.PageContext) : The context for the JSP page. Provides a single API to manage the various scoped attributes.
7. request(Subtype of javax.servlet.ServletRequest) : The request triggering the execution of the JSP page.
8. response(Subtype of javax.servlet.ServletResponse) : The response to be returned to the client. Not typically used by JSP page authors.
9. session(javax.servlet.http.HttpSession) : The session object for the client.
Explain the types of JSP actions.
- JSP actions are mainly XML tag which directs the server for using existing components or for controlling the behavior of the JSP engine.
- JSP Actions mainly comprises of a typical (XML-based) prefix of "jsp" followed by a colon ( : ), following by the action name & followed by one or more attribute parameters.
- The six JSP Actions are:
1. <jsp:include/>
2. <jsp:forward/>
3. <jsp:plugin/>
4. <jsp:usebean/>
5. <jsp:setProperty/>
6. <jsp:getProperty/>
When is class garbage collected?
- Java uses the garbage collector to free memory which is occupied by those objects which is no more reference by any of the program.
- An object becomes eligible for Garbage Collection when no live thread can access it.
- There are many ways to make a class reachable and thus prevent it from being eligible for Garbage Collection:
- 1. Objects of that class are still reachable.
2. Class object representing the class is still reachable.
3. ClassLoader that loaded the class is still reachable.
4. Other classes loaded by the ClassLoader are still reachable. - When all of the above are false, then the ClassLoader together with all classes it loaded are eligible for Garbage Collection.
Explain how a JSP is compiled into servlets by the container.
- JSPs are compiled into JavaServlets by the JSP container.
- A JSP compiler may generate a servlet in java code that is compiled by the java compiler or the bytecode may be generated directly for the servlet.
- JSP directives control the compilation process of a JSP that generates a servlet.
- Tomcat web server provides the JSP compilation as a task of ANT tool.
- The JSP compiled servlets are stored by ANT in work folder of CATALINA_HOME.
What is tag extension in JSP model?
- JSP supports the authors to add their own custom tags that perform custom actions.
- This is performed by using JSP tag extension API.
- A java class is written by developers which implements the tag interface and provides a tag library XML description file.
- This file specifies the tags and java classes that are used to implement the tags.
- The JSP tag extensions create new tags that can be inserted into the JavaServer Page directly.
- To write a code just extend SimpleTagSupport class and override the doTag() method, where the code can be placed to generate content for the tag.
What are the different types of enterprise beans exist?
- EJB uses the container to hold on the beans. There are two major types of bean that exist and they are as follows:
1. Stateful bean:- It consists of the business objects that call the client and deal with the session to access the client side session and their activities.
2. Stateless or Singleton bean:- It consists of business objects that have no association with one another.
- It allows a single bean instance to be associated with only one client at a particular time.
- This means that no concurrent processes can run at the same time and it is not allowed to be used.
- If any concurrent request is made for the processes then the container route the instance to another instance.
- This way the session can be thread safe and instance variables can be used.
Explain Struts.
- Struts is a open source software used to develop java based web page.
- Struts uses Jakarta Packages, Java Servlets, JavaBeans, ResourceBundles, and XML.
- Struts takes the help of Model View Controller (MVC) architecture.
- Where Model is referring to business or database, View is referring to the Page Design Code, and Controller is referring to navigational code.
What is Action Class?
- An Action class in the struts application is used to handle the request.
- It acts as interface or communication medium between the HTTP request coming to it and business logic used to develop the application.
- Action class consists of RequestProcessor which act as controller. This controller will choose the best action for each incoming request, generate the instance of that action and execute that action.
- This should be in thread-safe manner, because RequestProcessor uses the same instance for number of requests at the same time.
What are the classes used in Struts?
Struts Framework consists of following classes:- Action Servlets: Used to control the response for each incoming request.
- Action Class: Used to handle the request.
- Action Form: It is java bean, used to refer to forms and associated with action mapping.
- Action Mapping: Used for mapping between object and action.
- Action Forward: Used to forward the result from controller to destination.
What is MVC?
Model View Controller (MVC) is a design pattern used to perform changes in the application.
Model: - Model is referring to business or database.
- It stores the state of the application.
- Model has no knowledge of the View and Controller components.
View:- View is referring to the Page Design Code.
- It is responsible for showing the result of the user’s query.
- View modifies itself when any changes in the model happen.
Controller:- Controller is referring to navigational code.
- Controller will chose the best action for each incoming request, generate the instance of that action and execute that action.
What are the various Struts tag libraries?
The various Struts tag libraries are:- HTML Tags: Used to create the struts input forms and GUI of web page.
- Bean Tags:Used to access bean and their properties.
- Logic Tags: Used to perform the logical operation like comparison.
- Template Tags: Used to change the layout and view.
- Nested Tags: Used to perform the nested functionality.
- Tiles Tags: Used to manage the tiles of the application.
What is Jakarta Struts Framework?
- It is an open source framework for creating web applications
- Web applications, some deliver static content and some create dynamic response.
- A web application can perform database actions and perform business logic to make responses customized.
- A web application that uses JSP sometimes mix the operations like database code, design code, and control code. In this way a web application becomes difficult to maintain.
- Model, View, Control (MVC) architecture is one of the ways to separate the concerns in developing software applications.
- The struts framework is designed to isolate these process (MVC architecture) while designing web applications.
- The struts framework provides the key components for a web applicatons such as:
1. A
“request” is being handled by the request handler which is developed by application developer which is mapped to a standard URI.
2. A
“response” handler transferring the control from one resource to another that finishes the process of response.
3. A
"tag library" which is used to create interactive form-based applications by the developers with server pages.
- Struts also works well with the conventional applications with SOAP and AJAX.
What is Hibernate?
- Hibernate is an ORM tool. It is a solution for object-relational mapping and a persistence management layer.
- It is a powerful, high performance object/relational persistence and query service.
- It allows expressing queries in its own portable SQL extension (HQL), as well as in native SQL, or with an object-oriented Criteria and Example API.
- For example a java application is used to save data of an object to a database.
- Hibernate provides a solution for this persistence by mapping database tables to a class.
- The database data is copied to the object. The copying of data as objects and vice versa is known as object-relational mapping.
- A query that uses select statement and its operations of fetching tuples is done by a simple query object.
- And being an object, the same object can be utilized several times, which reduces the ‘query defining time’.
- Usage of simple java classes [Plain Old Java Objects ] makes this task simple instead of using entity beans / JDBC calls.