Android Adapter with Spinner Control
Describe adapter in Android.
Adapter objects in android work as a bridge between DataSource (Array, ArrayList, etc) and AdapterView. Some of the important objects of AdapterView are Spinner, ListView and GridView.
Android’s common adapters are:- ArrayAdapter
- CursorAdapter
- CursorAdapter
- BaseAdapter
- ListAdapter
How will you use spinner View with array. Explain with example.
Spinner control is same as dropdown list. You can select one item at a time from the spinner control.
Create an array in App → res → values → strings.xml file.
<resources>
<string name = "app_name">SpinnerDemo</string>
<string name = "action_settings">Settings</string>
<string-array name = "Days">
<item>Sunday</item>
<item>Monday</item>
<item>Tuesday</item>
<item>Wednesday</item>
<item>Thursday</item>
<item>Friday</item>
<item>Saturday</item>
</string-array>
</resources>
Following is the content of the modified content_main.xml file
<?xml version = "1.0" encoding = "utf-8"?>
<RelativeLayoutxmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app = "http://schemas.android.com/apk/res-auto"
xmlns:tools = "http://schemas.android.com/tools"
android:layout_width = "match_parent"
android:layout_height = "match_parent"
android:paddingBottom = "@dimen/activity_vertical_margin"
android:paddingLeft = "@dimen/activity_horizontal_margin"
android:paddingRight = "@dimen/activity_horizontal_margin"
android:paddingTop = "@dimen/activity_vertical_margin"
app:layout_behavior = "@string/appbar_scrolling_view_behavior"
tools:context = "com.example.custom.spinnerdemo.MainActivity"
tools:showIn = "@layout/activity_main">
<Spinner
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:id = "@+id/spinner"
android:layout_alignParentTop = "true"
android:layout_alignParentLeft = "true"
android:layout_alignParentStart = "true" />
</RelativeLayout>
Following is the content of the modified MainActivity.java file
public class MainActivityextends AppCompatActivity
{
Spinner spinner;
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
spinner = (Spinner) findViewById(R.id.spinner);
ArrayAdapter adapter = ArrayAdapter.createFromResource(this,
R.array.Days, android.R.layout.simple_spinner_item);
spinner.setAdapter(adapter);
spinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener()
{
@Override
public void onItemSelected (AdapterView<?> parent, View view, intposition, long id)
{
TextView txt = (TextView)view;
Toast toast = Toast.makeText(getApplicationContext(), "Selected day ="+txt.getText(), Toast.LENGTH_LONG);
toast.setGravity(Gravity.CENTER,0,0);
toast.show();
}
@Override
public void onNothingSelected (AdapterView> parent)
{
}
});
}
}
Run the application. The output will be like the image given below.
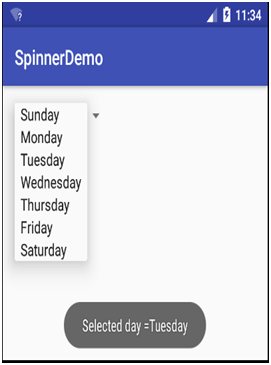