Give difference between GET and POST methods.
Sr. No. | GET Method | POST Method |
---|
1. | It is less secure. | It is more secure than GET method. |
2. | GET method variables are displayed in the URL. | POST method variables are not displayed in the URL. |
3. | It should not be used when sending passwords or other sensitive information. | It is used when sending passwords or other sensitive information. |
4. | URL length is restricted. | URL length is not restricted. |
5. | Only ASCII characters allowed. | No restrictions, binary data are also allowed. |
6. | Using this method, it is easy to hack the scripts. | Using this method, it is difficult to hack the scripts. |
7. | It can be bookmarked. | It cannot be bookmarked. |
8. | The parameters remain in the browser history because they are part of the URL. | The parameters not saved in the browser history. |
9. | It can be cached. | It cannot be cached. |
10. | It requests data from the specified resource. | It submits the data to be processed to a specified resource. |
How to declare array in PHP? Write PHP code to demonstrate array.
What is an Array?- It is a special variable that holds more than one value at a time.
- It is a group of objects with the same attributes.
- It is the arrangement of memory cells in the RAM.
- Each object in an array is called an array element.
- It is a systematic arrangement of similar objects, usually in rows and columns.
What is PHP?- PHP stands for Hypertext Preprocessor.
- It is a server-side scripting language, mostly designed for web development but also used as a general-purpose programming language.
- It is a widely used open source general-purpose scripting language which is especially suited for web development and can be embedded into HTML.
How to declare array in PHP?
The array() function is used for creating an array in PHP.
There are three types of arrays in PHP:
1. Indexed Array
2. Associative Array
3. Multidimensional Array
1. Indexed Array
There are two ways to create indexed arrays:
I. Automatically
II. Manually
I. Automatically:
The index can be assigned automatically.
Example:
$fruits = array(“apple”, “mango”, “orange”);
II. Manually:
The index can be assigned manually.
Example:
$fruits[0] = “apple”
$fruits[1] = “mango”
$fruits[2] = “orange”
In the above example, it creates an indexed array named $fruits. The $fruits array assigns three elements to it and then prints a text containing the array values.
Example of Indexed Array:
<html>
<body>
<?php
$fruits = array("apple", "mango", "orange");
echo "I like " . $fruits[0] . ", " . $fruits[1] . " and " . $fruits[2] . ".";
?>
</body>
</html>
Output:
I like apple, mango, orange.
2. Associative Array
These arrays use named keys.
There are two ways to create an associative array:
I. $quantity = array(“apple”=> “10”, “mango”=> “5”, “orange”=> “10”);
II. $quantity['apple'] = “10”;
$quantity['mango'] = “5”;
$quantity['orange'] = “10”;
Example of Associative Array:
<html>
<body>
<?php
$ quantity = array(“apple”=> “10”, “mango”=> “5”, “orange”=> “10”);
echo "Quantity of Apple is " . $quantity['apple'] . " 10.";
echo "Quantity of Mango is " . $quantity['mango'] . " 5.";
echo "Quantity of Orange is " . $quantity['orange'] . " 10.";
?>
</body>
</html>
Output:
Quantity of Apple is 10.
Quantity of Mango is 5.
Quantity of Orange is 10.
3. Multidimensional Array- It can be stored in multidimensional arrays.
- It contains one or more arrays.
- PHP understands multidimensional arrays that are two, three, four, five, or more levels deep.
$fruits = array
(
array(“apple”,10,20),
array(“mango”,5,25),
array(“orange”,10,20)
);
Example of Multidimensional Array:
<html>
<body>
<?php
$fruits = array
(
array(“apple”,10,20),
array(“mango”,5,25),
array(“orange”,10,20)
);
echo $fruits[0][0].": In stock: ".$fruits[0][1].", sold: ".$fruits[0][2].".<br>";
echo $fruits[1][0].": In stock: ".$fruits[1][1].", sold: ".$fruits[1][2].".<br>";
echo $fruits[2][0].": In stock: ".$fruits[2][1].", sold: ".$fruits[2][2].".<br>";
?>
</body>
</html>
Output:
apple: In stock: 10, sold: 20.
mango: In stock: 5, sold: 25.
orange: In stock: 10, sold: 20.
Discuss features of PHP.
What is PHP?- PHP stands for Hypertext Preprocessor.
- It is a server-side scripting language, mostly designed for web development but also used as a general-purpose programming language.
- It is a widely used open source general-purpose scripting language which is especially suited for web development and can be embedded into HTML.
- PHP is integrated with a number of popular databases, including MySQL, Oracle, Sybase, Microsoft SQL Server.
- It supports a large number of major protocols such as POP3, IAMP and LDAP.
Features of PHP:- It provides cross platform compatibility, unlike some other server side scripting language.
- It includes variable variables; that means the name of the variable can be changed dynamically.
- It contains access monitoring capability to create logs as the summary of recent accesses.
- It includes several magic methods that begin with '_' and character which will be defined and called at appropriate instance. For example, _clone() will be called, when the 'clone' keyword is used.
- It supports extended regular expression that leads extensive pattern matching with remarkable speed.
- It is a single inheritance language, the parent class methods can be derived by only one directly inherited sub class.
- It has set of pre-defined variables called superglobals, which will be the start by '_'. For example, $_POST, $_GET etc.
- There is no need to specify the data type for variable declaration. It can be determined at the time of execution depends on the value of the variable. So PHP is called as loosely-typed language.
Uses of PHP:- It performs system functions, that is, from files on a system it can create, open, read, write and close them.
- It can handle forms, that gathers it data from files, save data to a file, through email, you can send data, return data to the user.
- Using PHP, add, delete, modify elements are used within the database.
- It accesses cookie variables and set cookies.
- PHP can encrypt data.
- It can restrict users to access some pages of the website.
Example:
<html>
<head>
<title>Welcome!!!</title>
</head>
<body>
<?php echo "Welcome!!!";?>
</body>
</html>
Output:
Welcome!!!
What is difference between $message and $$message?
Sr. No. | $message | $$message |
---|
1. | It is used to store variable data. | It can be used to store variable of a variable. |
2. | Data stored in $message is fixed. | Data stored in $$message can be changed dynamically. |
3. | It does not allow the developer to change the name of the variable. | It allows the developer to change the name of the variable. |
4. | Example:
$var1 = 'variable 1' | Example:
$$var1 = 'variable 2' |
5. | It is a simple variable. | It is a reference variable. |
Example:
$Message = "You";
$you= "Me";
echo $message //Output : You
echo $$message //Output : Me
- $message is a variable with a fixed name. $$message is a variable whose name is stored in $message.
- If $message contains 'var', then $$message is the same as $var.
- They both are variables. $$message is a variable whose name is stored in $message.
Write PHP Code to accept & display voter details from voter. After successful insertion display voter details in proper format (Assume suitable structure).
voter-details-add.html
<html>
<body>
<h1><u>Registration Form</u></h1>
<form action="voter-details.php">
<table border="0" width="30%" height="20%">
<tr>
<td>Name:</td>
<td><input type="text" name="vname"></td>
</tr>
<tr>
<td>Date of Birth(YYYY-MM-DD):</td>
<td><input type="text" name="vdob"></td>
</tr>
<tr>
<td>City:</td>
<td><input type="text" name="vcity"></td>
</tr>
<tr>
<td>State:</td>
<td><input type="text" name="vstate"></td>
</tr>
<tr>
<td colspan="2" align="center">
<input type="submit" value="Save"></td>
</tr>
</form>
</body>
</html>
voter-details.php
<?php
$con = mysql_connect("localhost","root","mysql") or die(mysql_error());
mysql_select_db("voters",$con) or die(mysql_error());
$vname = $_REQUEST["vname"];
$vdob = $_REQUEST["vdob"];
$vcity = $_REQUEST["vcity"];
$vstate = $_REQUEST["vstate"];
$insert_query = "INSERT INTO voters(vname,vdob,vcity,vstate) VALUES(' ".$vname." ',' ".$vdob." ',' ".$vcity." ',' ".$vstate." ')";
//Execute the query on the database
mysql_query($insert_query,$con) or die(mysql_error());
echo "<h3>Voter details have been saved successfully !!</h3>";
// Displaying details
$select_qry = "SELECT * FROM voters";
echo "<table border = 1><tr><th>Voter ID</th><th>Name</th><th>Birthdate</th><th>City</th><th>State</th></tr>";
$result = mysql_query($select_qry,$con) or die(mysql_error());
while($row=mysql_fetch_array($result))
{
echo "<tr><td>".$row['vid']."</td><td>".$row['vname']."</td><td>".$row['vdob']."</td><td>". $row['vcity']."</td><td>".$row['vstate']."</td></tr>";
}
//close the database connection
mysql_close($con);
?>
Output:
voter-details-add.html
voter-details.php
voters Table
Voter ID | Name | Birthdate | City | State |
1 | ABC | 10-05-1990 | Pune | Maharashtra |
Write PHP Code to display list of senior citizens from citizen table. (Assume suitable table structure).
Citizen Table:
Name | Age | Place |
---|
ABC | 55 | Pune |
XYZ | 59 | Mumbai |
PQR | 68 | Kolhapur |
senior-citizen.php
<html>
<body>
<h1><u>Details of Senior Citizen </u></h1>
<?php
$con = mysql_connect("localhost","root","mysql")or die(mysql_error());
mysql_select_db("Citizen",$con) or die(mysql_error());
$result = mysql_query("SELECT * FROM Citizen WHERE age>=60");
echo "<center><table border=1><tr><th>Name</th><th>Age</th><th>Place</th></tr></center>";
while($row=mysql_fetch_array($result))
{
echo "<tr><td>".$row['Name']."</td><td>".$row['age']."</td><td>".$row['place']."</td></tr>";
}
mysql_close($con);
?>
</body>
</html>
Output:
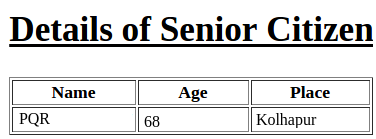