Q.8) Write short notes on (Any Two):
a) Friend function and Friend Class.
b) Stream based I/O in C++.
c) Exception Handling Mechanism.a) Friend function and Friend Class.Ans.
friend FunctionIf a function is defined as a friend function then, it can access the private and protecteddata of that class.
class class_name
{
-------
friend return_type function_name(argument_list);
-------
}
Friend ClassIf object of one class wants to access the private data member and member function of other class then we can declare a class as friend class. C++ provides a keyword friend, by using this keyword you can declare a class or function as friend. Friend function or friend class should be used carefully because it hit the encapsulation concepts.
The syntax of creating a class as friend is as follows.
friend class class_NameImportant points regarding friend class.-Friendship is not mutual. If a class A is friend of B, then B doesn’t become friend of A automatically.
-Friendship is not inherited.
b.)Stream based I/O in C++.
Ans.To implement input/output operations, C++ uses stream and stream classes. This I/O system handles the wide variety of devices such as terminals, disks, and tape drives. These devices are made by different companies, so C++ I/O system provides an interface that is independent of I/O devices. This interface is called as stream.
A stream is a sequence of bytes or character. This stream can works as either source ordestination for devices. Source stream that provides the data is called as input stream and the stream that receives the data is called as output stream. Input stream can receive data from keyboard or other devices and output stream provides the data to the screen.
C++ stream classesI/O system of C++ defines different classes to define various streams. These classes deals with console and disk files. These classes are known as stream classes.
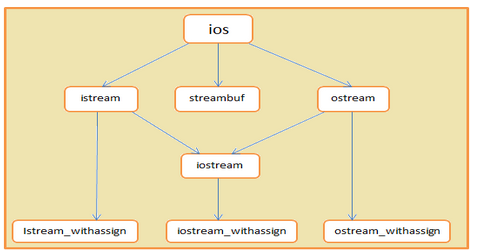
IOS is the most base class of the above given class hierarchy. It is the base class of istream, ostream class. IOS is the virtual base class so that only one copy of public and protected members are inherited in derived class. IOS class provides the basic functionality and used by other input and output classes.
Istream class represent the input stream and provides the input function such as get(), getline(), and read().
ostream class represent the output stream and provides the output function such as put() and write().
c.) Exception Handling Mechanism.
Ans.In C++ there are three keywords for exception handling and those keywords are try, catch, and throw. try block contains you main code that might potentially throw an exception. If an exception occurs within the
try block, it is thrown by using
throw keyword. Exceptions that are thrown by try block are caught by a
catch block.There can be more than one catch statement associated with a
try block. Which catch block will be executed, it will be depend upon the type of the exception.If there is no corresponding catch block is available then you program will abnormally terminated.
If there is no error occurs within the
try block, then no
catch statement is executed.
Output:Type of exception is integer
Type of exception is double
Catching all exceptionsIn some situations you are not able to understand that which type of exception will occur. In such situation you need a catch block that can catch all type of exception. This could be achieved by declaring catch block as follows.
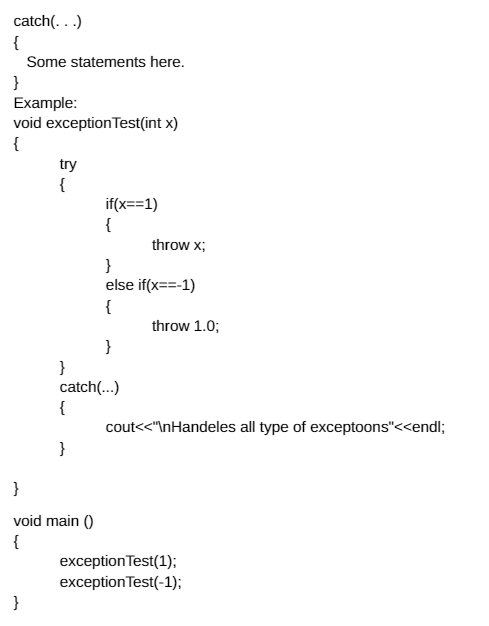