Java multithreading tutorial - contributed by Pradip Patil
Multithreading
Two or more parts of the program that can run concurrently is known as
multithread program. Each part of this program is called thread.
Multi-threading is a special form of multitasking. Multi-threading allows you
to write very efficient program that makes maximum use of CPU because idle time
is kept to minimum.
Thread: Java program runs with the thread known as main thread.
In java there are 2 ways to create thread
1)declare a class to be a subclass of thread.
Class Demo extends Thread
{
Demo()
{ start() ;)
Public void run(){ }
}
Start this thread like
Demo d=new Demo();
2) declared class that implements runnable interface.
Class Demo implements Runnable
{
Thread t;
Demo()
{
t=new
Thread(this,”Second”);
t.start();
}
Public void run(){ }
}
Both class as well as interface consists of run method
Thread Life cycle
Like life cycle of a creature on the earth, threads also have
life cycle. In that life cycle thread goes through different states viz.
1)new/ born state
2)runnable state
3)Not runnable/blocked state
4)Dead state
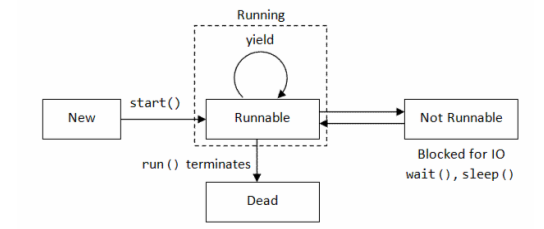
These are the four states of the thread life cycle
1)new/born: in this state, start() method is called.
2)runnable : yield () is used.
3)not Runnable/Blocked : Wait() or sleep method is used.
4)Dead : when run method is complete it goes to dead state.
Thread priority: Thread priority shows how thread is treated
with respect to others at the time of execution. Priority is in integer and
priority is meaningless if it is the only thread running. Thread priority is
decided at the time of context switching.
Priority is in between Thread.MAX_PRIORITY and
Thread.MIN_PRIORITY.
MAX_PRIORITY: thread have maximum priority with value 10.
MIN_PRIORITY : Minimum priority that thread have value 1.
NORM_PRIORITY: Default priority assign to thread, value is 5.
Thread Synchronization : Excluding the other threads from an
operation until the operation is complete is called synchronization.
There are 2 ways to synchronize thread code execution
1)Synchronize block of the code
// File Name : Demo.java
// This program uses a synchronized block.
class Demo
{
void Demo(String msg)
{
System.out.print("[" + msg);
try
{
Thread.sleep(1000);
} catch (InterruptedException e)
{
System.out.println("Interrupted");
}
System.out.println("]");
}
}
// File Name : Demo1.java
class Demo1 implements Runnable
{
String msg;
Demo target;
Thread t;
public Demo1(Demo targ, String s)
{
target = targ;
msg = s;
t = new Thread(this);
t.start();
}
// synchronize calls to call()
public void run()
{
synchronized(target) { // synchronized block
target.call(msg);
}
}
}
// File Name : Sample.java
class Sample
{
public static void main(String
args[])
{
Demo target
= new Demo();
Demo1 ob1 =
new Demo1(target, "Hello");
Demo1 ob2 = new
Demo1(target, "Synchronized");
Demo1 ob3 = new
Demo1(target, "Java");
// wait for threads to end
try {
ob1.t.join();
ob2.t.join();
ob3.t.join();
}
catch(InterruptedException e) {
System.out.println("Interrupted");
}
}
}
2)synchronized method
class Demo extends Thread
{
static String msg[]={"This", "is",
"a", "synchronized", "variable"};
Demo(String threadname){
super(threadname);
}
public void run()
{
display(getName());
}
public synchronized void display(String threadN)
{
for(int i=0;i<=4;i++)
System.out.println(threadN+msg[i]);
try{
this.sleep(1000);
}catch(Exception e){}
}
}
public class Sample
{
public static void
main(String[] args)
{
Demo t1=new Demo("Thread One: ");
t1.start();
Demo t2=new Demo("Thread Two: ");
t2.start();
}
}
Inter thread communication
In thread, inter process communication is very important to avoid the polling .
Polling means to check some conditions repeatedly, once condition is true then
take appropriate action .But to avoid polling, inter process communication
mechanism is used. It consists of wait(), notify(), notifyAll() methods. These
methods are implemented as final method in Object. So all classes have them.
|