RMI tutorial - contributed by Pradip Patil
RMI
Introduction & Architecture of RMI
Remote Method Invocation allows applications to call object methods located
remotely, sharing resources and processing load across systems. Client
information is transferred into intermediate format like XML or HTML and
parsing of that data is done by the server. RMI application consists of two
separate programs known as client and server.
RMI based on earlier technology for procedure programming is called remote
procedure calls (RPC). RPC performs all networking and marshalling of the
data..
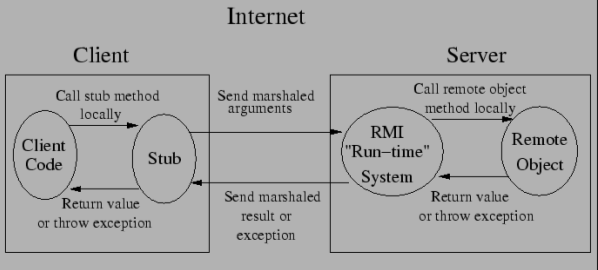
RMI Architecture
The Stub/Skeleton: Stubs are detailed to the client side,
whereas skeletons are initiated on the server side. To achieve the transparency
about location, RMI introduces two objects known as stubs and skeletons.
Purpose of this is to transfer data to server and client to perform the
marshalling and unmarshalling .
Marshalling is the process of converting the data or object being transferred
into a byte stream and unmarshalling is the reverse of marshalling i.e –
converting the stream into an object or data. This conversion is achieved via
serialization of the object.
Stub
Events performed by the stub:
-
Pledges a connection with the remote object of remote VM
-
Marshals the parameters to the remote VM.
-
Waits for the result of the method invocation.
-
Unmarshals the return value .
Skeleton
Events performed by the skeleton:
-
Unmarshals the parameters for the remote method .
-
Invokes the method on the actual remote object implementation.
-
Marshals the result .
RMI classes and interfaces:
All classes and method hierarchy of the RMI is available in the java.rmi
package.
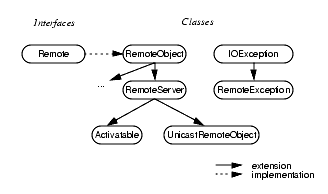
1) java.rmi.Remote Interface: A remote interface is an interface that declares a
set of methods that may be invoked from a remote Java virtual machine.
Java.rmi.Remote interface have no methods.
Eg:
Following Demo in interface is remote interface
public interface Demo extends java.rmi.Remote
{
public void display() throws
java.rmi.RemoteException;
}
2) The RemoteException Class:The java.rmi.RemoteException class is the
superclass of exceptions thrown by the RMI runtime during a remote method
invocation. It is a checked exception - not a runtime exception.
3) The RemoteObjectClass :RMI server functions are provided by
java.rmi.server.RemoteObject and its subclasses.
Writing simple RMI Application:
Using RMI to develop a distributed application contains these steps:
1. Designing and implementing the components of your distributed application.
2. Compiling sources.
3. Making classes network accessible.
4. Starting the application.
Designing Remote interface
import java.rmi.*;
import java.rmi.server.*;
public interface ArithRemote extends Remote
{
public int add(int a,int b) throws RemoteException;
public int subtract(int a,int b) throws RemoteException;
public double multiply(int a,int b) throws RemoteException;
public float divide(int a,int b) throws RemoteException;
}
Implementing the remote interface
import java.rmi.*;
import java.rmi.server.*;
public class ImplArithRemote extends UnicastRemoteObject implements ArithRemote
{
public ImplArithRemote() throws RemoteException
{}
public int add(int a,int b)
{
return(a+b);
}
public int subtract(int a,int b)
{
return(a-b);
}
public double multiply(int a,int b)
{
return(a*b);
}
public float divide(int a,int b)
{
return(a/b);
}
}
Client
import java.rmi.*;
public class ArithClient
{
public static void main(String arg[])
{
if(System.getSecurityManager()==null)
{
System.setSecurityManager(new RMISecurityManager());
}
try
{
ArithRemote o=(ArithRemote)Naming.lookup("/MyObj");
String str=String.valueOf(o.add(5,6));
System.out.println("Sum="+str);
str=String.valueOf(o.subtract(10,3));
System.out.println("Subtract="+str);
str=String.valueOf(o.multiply(5,6));
System.out.println("Multiply="+str);
str=String.valueOf(o.divide(6,2));
System.out.println("Divide="+str);
}catch(Exception
e)
{
System.out.println("Exception "+e);
}
}
}
RMI Server
import java.rmi.*;
public class ArithServer
{
public static void main(String arg[])
{
try
{
ImplArithRemote obj=new ImplArithRemote();
Naming.rebind("/MyObj",obj);
System.out.println("Server has started.....");
}catch(RemoteException e)
{
System.out.println("Exception "+e);
}catch(Exception e)
{
System.out.println("Exception "+e);
}
}
}
Parameter passing in remote methods: A remote object can be
any object that is serializable
It consists of primitive types, remote objects, and non-remote objects that
implement the java.io.Serializable interface. Converting data into a format
that can be used either for storage or for transmission to another process is
called marshalling . Unmarshalling involves taking the converted data and
recreating the objects .
|