What Is ASP.NET AJAX?
The ASP.NET Component
The JavaScript Component
The Web Services Component
The Dynamic HTML Component
What Is ASP.NET AJAX?
ASP.NET AJAX is the name of Microsoft’s AJAX solution, and it refers to a set of
client and server technologies that focus on improving web development with
Visual Studio. Other companies have their own AJAX solution, often taking a
radically different approach, but Microsoft has sought to build upon the
popular ASP.NET technology by developing a comprehensive set of script
libraries as well as server programming enhancements. One piece, the Microsoft
AJAX Library, is a JavaScript library that works on a variety of browsers and
serves to simplify JavaScript development. It provides you with the capability
to easily write JavaScript using an object-oriented programming approach
similar to code-behind programming in ASP.NET. You can use the functions of the
Microsoft AJAX library to interact with the DOM, dynamically update portions of
the page, communicate with the web server asynchronously, and write rich
animations on the page. You’ll learn about the client features in Chapters 6
and 7, but for now think of the Microsoft AJAX Library as a type system and set
of class libraries that simplify writing JavaScript to enhance the user
experience, manipulate the DOM, and communicate with the web server. The huge
benefit in using this library is that it abstracts the tedious low-level DHTML
programming into an OOP model that is much easier to work with.
|
Book Excerpt: Developing Next-Generation Web Applications
Chapter Contents
This excerpt from
Professional ASP.NET 2.0 AJAX by Matt Gibbs, Dan Wahlin, is
printed with
permission from Wrox Publication.
|
The other part of the ASP.NET AJAX release is the server-side ASP.NET 2.0 AJAX
Extensions. These extensions build on top of the ASP.NET classes and controls
and leverage the Microsoft AJAX Library sent to the browser. They make it easy
to quickly take advantage of AJAX technologies to enrich an application.
Through a set of standard web services, ASP.NET AJAX is also able to leverage
server-based application services such as authentication and user profile
storage. The extensions both extend some of the existing ASP.NET controls by
adding new behaviors and include a new set of server controls that make it easy
to do asynchronous updates to a page and leverage server resources.
The client and server pieces of ASP.NET are shown in Figure 1-3. ASP.NET is
built on top of the Microsoft Internet Information Services (IIS) web server.
ASP.NET AJAX builds on top of that and the web services it includes. The
Microsoft AJAX Library runs in the browser to manipulate the DOM, communicate
asynchronously with the web server, and take advantage of ASP.NET services.
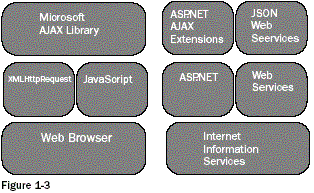
The ASP.NET Component
The original (ASP) Active Server Pages technology was released as part of
Internet Information Server 3.0. It was then improved with support for
transactions and access to COM objects with the release of the NT 4.0 Option
Pack almost ten years ago. At the time, most websites consisted of static HTML
pages. To the extent that there were any dynamic applications, they were CGI
(Common Gateway Interface) or ISAPI (Internet Server API) applications
typically written in C and C++. With the release of Active Server Pages (ASP),
developers could use JavaScript or VBScript on the server to leverage a set of
intrinsic objects provided by ASP. “Classic ASP,” as it is now called, provided
a session object so that developers didn’t need to worry about the stateless
nature of HTTP. It had Request and Response objects that provided for easy
access to data coming from forms on the client and a way to send updated
information back. The Server and Application objects were an avenue for
accessing information from the web server and utilizing a common set of COM
objects across the application.
Although Classic ASP was a big win for developers, developers soon found that
developing complex applications became difficult. The lack of support for
modularization meant that applications ended up with such complex
interdependencies in script that developers often referred to it as “spaghetti
code.” Debugging support was severely lacking, and there were no rich design
tools for developing applications. Performance also suffered because ASP used a
slow scripting model, and syntax errors could only be detected at runtime.
ASP.NET is a big leap forward. It provides compatibility for existing
applications by including the same set of intrinsic objects found in Classic
ASP but moves from interpreting JavaScript and VBScript on the fly to using a
compiled set of pages and modules written in C# and VB.NET. Classic ASP simply
executed script in order from the top to the bottom of the page; ASP.NET is an
event-driven model with a page lifecycle, making it more like writing a desktop
application. Now, instead of including separate JavaScript files to represent
business objects, you can create objects in any .NET language and access them
directly from ASP.NET.
ASP.NET takes a set of pages that contain code and markup and generates a Page
class that is then compiled and cached. For each request to the page, the class
is instantiated and a complete page lifecycle is executed. A set of events are
executed, some of which have been overridden by the generated Page class.
Controls in the page also participate in the lifecycle, databinding to backend
databases, reacting to user input, and dealing with changes to their state from
the user’s previous view. To react to user actions, the developer has only to
provide an event handler for the given action. For example, the button control
exposes a Click event. When using it, you don’t need to write code to examine
all form variables on a page to know if the button was clicked. Instead, you
just provide code for an event handler override. The event handler code can
then update the HTML for the page or the properties and data of other controls
on the page.
The JavaScript Component
AJAX technologies take advantage of the common support for JavaScript found in
modern browsers. Because there is a standard that is supported across the
various browsers, you can write scripts knowing that they will run. This wasn’t
always the case.
In the mid 1990’s, Netscape and Microsoft (along with others) collaborated on a
standard for a scripting language that they would support in their web
browsers. The standard is called EcmaScript. Microsoft’s implementation is
called JScript, but the language is generally referred to as JavaScript, as it
was called in Netscape. (It has nothing to do with Java, but someone must have
thought the association was useful for marketing purposes.) JavaScript program
snippets are sent down to the browser along with the HTML, and they run inside
the user’s browser to affect how the page is processed on the client.
JavaScript is not compiled; it is interpreted. There is no static type-checking
like you get in C++ and C#. You can declare a variable without needing to
specify a type, and the type to which the variable refers can change at any
time. This makes it easy to get started programming in JavaScript, but there’s
inevitably a certain amount of danger in allowing the data type of a variable
to change dynamically at runtime. In the following snippet, notice that the
variable can reference any type without difficulty:
var something = 1;
something = true;
something = “a string”;
JavaScript is a dynamic language. Types can actually be extended during program
execution by other code. This means that you can write code that creates types
on the fly. Because there is no enforcement of type safety, your code can
receive these types as parameters or return values without any problem. This
provides a great degree of flexibility and coding power.
The fundamental types in JavaScript are strings, numbers, Booleans, and
functions. There is also support for objects and arrays, which are collections
of the fundamental types. Some additional objects are included that are
considered essential for many programming tasks. This includes support for
regular expressions and date and time operations.
You can use the plus operator on strings in JavaScript to concatenate them:
var theEnd = “THE END.”;
var result = “Beginning, “ + “middle, and “ + theEnd;
In this example, the result variable is now the string: “Beginning, middle, and
THE END.”
JavaScript interpreters use the IEEE floating-point standard for storing
numbers. Ignoring the gory details, you can assume that for most programming
tasks you won’t have any trouble.
The Boolean type in JavaScript is about what you would expect it to be but maybe
not exactly so. The Boolean represents whether or not an expression is true,
but it uses the C-style convention using integer values 0 and 1.
Variables can exist in JavaScript without having a value, and a variable may
simply be undefined, which can produce unexpected results. In this piece of
JavaScript, three variables are declared, and all of these comparisons are
designed to return a true value.
<script type=”text/javascript”>
var one = 1;
var zero = 0;
var undefinedVar;
if(one)
{
alert(“1 is true”);
}
if(!zero)
{
alert(“0 is false”);
}
if(!undefinedVar)
{
// this test tells us that “undefinedVar”
either contains 0,
// or is really undefined: both of
which equate to false
alert(“undefinedVar is false”);
}
if(one != zero)
{
alert(“one and zero are not the same”);
}
</script>
You can check specifically to see if a variable has been defined like this:
if( typeof(undefinedVar ) == “undefined” )
{
alert(“undefinedVar is undefined”);
}
Variables can also have a null value, which is not the same thing as being
undefined, as a null value does constitute a value.
Functions are also real types in JavaScript. They can accept arguments and
return values. Functions can be passed to other functions and can be created
dynamically by other script code.
Here are two equivalent definitions for a function called Add that will take two
variables and return the result of applying the plus operator. Notice that I
didn’t state that it takes two numbers. Remember, JavaScript variables don’t
have a defined type, so I could just as easily pass two strings and get them
concatenated by my Add function.
<script type=”text/javascript”>
function Add(x, y)
{
return x + y;
}
var AddAgain = function(x, y) { return x + y; }
</script>
Once either of these styles is used to create a function, it can be called from
that scope and any nested scope to perform the addition. There is no advantage
to one of these forms over the other. You can simply choose to use the syntax
that you prefer.
<script type=”text/javascript”>
var result = Add(36, 24);
alert(result); //displays 60
var stringResult = Add(“Hello “, “there.”);
alert(stringResult); //displays “Hello there.”
</script>
Objects and arrays are just collections of other types. Array types do not
require that the values they hold be named; instead, you can access them by
index. The values held in an object are referenced by field or property names.
Objects can also hold functions (which can be accessor functions to give public
visibility to local variables), which lets you create data structures that
represent entities in JavaScript code. Missing from this sort of
object-oriented programming is a concept of type inheritance. The Microsoft
AJAX Library provides a set of classes and recommended patterns for achieving
inheritance in JavaScript, making it more natural for switching between
JavaScript and other high-level languages. The following code example includes
a definition for an Album object that holds and returns the artist and album
title. An array is then used to store information about several albums.
<script type=”text/javascript”>
// define an object named Album – note that this object is typeless
Album = function(title, artist) {
var _title = title;
var _artist = artist;
this.get_title = function() { return _title; }
this.get_artist = function() { return _artist; }
}
// create object instances by calling the constructor
var albumA = new Album(“Rift”, “Phish”);
var albumB = new Album(“A Picture of Nectar”, “Phish”);
// create an array to hold the instances (also typeless)
var albumArray = new Array();
albumArray[0] = albumA;
albumArray[1] = albumB;
// iterate over the array to show the album titles
for(var i = 0; i < albumArray.length; i++) {
alert((albumArray[i]).get_title()); //
call get_title accessor
}
</script>
The Web Services Component
The fundamental concept of web services is powerful and continues to evolve and
advance. The original SOAP (Simple Object Access Protocol) standard is the use
of the HTTP protocol to pass XML-formatted data to the server from a client and
receive XML-formatted results in return. This can be from within a web browser
using the XmlHttpRequest object or directly from a desktop application or
another server. Before web services became widely adopted, it was not uncommon
for developers to programmatically request a page as an HTML document and
extract the desired data from it, a technique known as screen-scraping. This
causes all sorts of frustrations as sites are continually updated and the
screen-scraping clients must try to keep up by modifying their parsing code to
adapt to the new HTML the target site is rendering.
This produced frustration, as sites that presented data using HTML visual pages
were prone to modifying those pages and this would break the screen-scraping
program, which expected to see the data in the original format. Web services
were created as a nonvisual way to transfer data over the web, and they are the
natural way to isolate remote method calls from the presentation layer. Now,
instead of screenscraping, you are able to call a web service and get
XML-formatted data returned that is easily consumed by a program.
By passing plain text data formatted as XML and by eliminating the visual
elements, data passed in web services is much easier to parse than HTML. And,
since XML can contain an embedded schema, code can inspect the schema and use
it to determine the structure and types used in the data. You can extend the
schema passed with the data being returned without worrying that consuming
applications will be broken, and therefore XML readers can be somewhat tolerant
of modifications that would have certainly caused a screen-scraper a great deal
of grief!
The schema for data can be extended without requiring all consumers to be
updated. Consumers can easily get the parts of the XML document they wish to
process and disregard the rest. This has progressed beyond simple XML formats.
Unlike previous implementations of web services, you can now define web service
contracts to be built to employ arbitrary encoding and utilize any one of a
number of wire protocols. What drives the web service concept is the ability to
access data easily from various applications in a loosely coupled way, and the
new Microsoft Windows Communication Foundation (WCF) takes this concept to a
whole new level, allowing the contract to specify wire protocols, deployment
strategies, and logging infrastructure, along with providing support for
transactions.
ASP.NET AJAX provides a set of JavaScript proxy objects to access some new web
services built-in to ASP.NET. Profile information, membership services, and
role management can be easily accessed from the client. Developers don’t need
to create their own infrastructure to support these fundamental application
services, but can include a few lines of code to take advantage of server
resources from JavaScript code running in the browser, thereby dramatically
extending the reach of ASP.NET to include both the client and the server. And
because the JavaScript libraries are designed to be easy to use for developers
already familiar with server-side .NET programming, all of this extra
functionality comes in a friendly package that is easy to leverage.
The Dynamic HTML Component
Dynamic HTML is not a freestanding technology. It is the use of a set of
technologies in a specific way. HTML is returned to the browser following a web
server request. The browser then renders the page, and the user is able to view
it. The browser also exposes the Document Object Model (DOM) that represents
the structure of the HTML being displayed. The DOM can be accessed from
JavaScript embedded in, or referenced by, the page. The appearance of the HTML
is affected by applying CSS (Cascading Style Sheets) that control colors,
fonts, position, visibility, and more. You can bind JavaScript code to events
that the browser will raise when users perform certain actions, such as
hovering over a particular element or entering text in a textbox. The
JavaScript code can update text or manipulate the CSS settings for elements
within the page. And, of course, it can communicate with the server to expand
the dynamic nature of the page even further. The user will see a dynamically
changing user interface that responds to his actions in real-time, which will
greatly enhance his overall experience, thereby increasing his productivity and
satisfaction with the application.
More Related links
Answer - ASP.NET Ajax Framework is used for implementing the
Ajax functionality......
Answer - Back functionality cannot work because the dynamic
pages don’t register themselves to the browsers history engine..........
AJAX is an acronym for Asynchronous JavaScript and XML. If you think it doesn't
say much, we agree. Simply put, AJAX can be read "empowered JavaScript",
because it essentially offers a technique for client-side JavaScript to make
background server calls and retrieve additional data as needed, updating
certain portions of the page without causing full page reloads.............
With ASP.NET 2.0, things have changed for the better. For security-related
functionalities, ASP.NET 2.0 introduces a wide range of new
controls..............
In addition to the new controls, ASP.NET 2.0 also provides numerous enhancements
to existing controls that make these controls more versatile than ever before
in building component-based web pages. For example, the Panel control now has a
DefaultButton property that specifies which button should be clicked if the
user presses the Enter key while the panel has the focus.........
|