Book Excerpt: Object-Oriented JavaScript
|
|
This chapter excerpt from
Microsoft AJAX Library Essentials by Cristian Darie, Bogdan
Brinzarea, is printed with permission from Packt
Publishing, Copyright 2007.
Inheritance using Closures and Prototypes
There are two significant techniques for implementing the OOP concept of
inheritance with JavaScript code. The fi rst technique uses closures, and the
other technique makes use of a feature of the language named
prototyping.
Early implementations of the Microsoft AJAX library made use of closures-based
inheritance, and in the fi nal stage the code was rewritten to use prototypes.
In the following few pages we'll quickly discuss both techniques.
|
Inheritance Using Closures
In classic OOP languages such as C#, C++, or Java, you can extend classes
through inheritance. Closure-based inheritance is implemented by creating a
member in the derived class that references the base class, and calling that
member. This causes the derived class to inherit all the base class members,
effectively implementing the concept of inheritance.
To demonstrate this technique, we'll implement two classes: Car and SuperCar.
The Car class constructor receives a car name as parameter, and it has a method
named Drive(). The class SuperCar inherits the functionality of Car, and adds a
new method named Fly(), reflecting the additional functionality it has in
addition to what Car has to offer. The diagram in Figure 3-6 describes these
two classes.
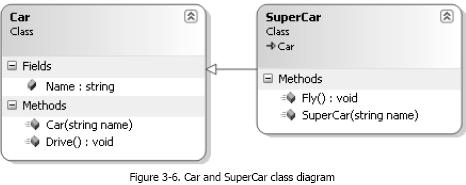
Rember that in JavaScript the implementation of a class diagram can be achieved
in multiple ways. The code refl ects the concept of the diagram, but not also
the implementation details, as the C# code would. Here's a possible
implementation of Car and SuperCar:
<script type="text/javascript">
// to be used as the Drive method of Car
function Drive()
{
document.write("My name is " + this.Name +
" and I'm driving. <br />");
}
// class Car
function Car(name)
{
// create the Name property
this.Name = name;
// Car knows how to drive
this.Drive = Drive;
}
// to be used as the Fly method of SuperCar
this.Fly = function()
{
document.write("My name is " + this.Name + " and I'm flying! <br />");
}
// class SuperCar
function SuperCar(name)
{
// implement closure inheritance
this.inheritsFrom = Car;
this.inheritsFrom(name);
// SuperCar knows how to fly
this.Fly = Fly;
}
// create a new Car and then Drive
var myCar = new Car("Car");
myCar.Drive();
// create SuperCar object
var mySuperCar = new SuperCar("SuperCar");
// SuperCar knows how to drive
mySuperCar.Drive();
// SuperCar knows how to fly
mySuperCar.Fly();
</script>
Loading this script in a browser would generate the results shown in Figure 3-7.
It can be tested online at
http://www.cristiandarie.ro/asp-ajax/JavaScriptClosureInheritance.html.
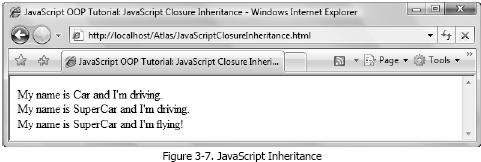
The exercise demonstrates that inheritance really works. SuperCar only defi nes
the capability to Fly(), yet it can Drive() as well. The capability to Drive()
and the Name prop erty are inherited from Car.
At the fi rst sight the code can look a bit complicated, especially if you're a
C# veteran. The Drive() and Fly() functions aren't defi ned inside Car and
SuperCar, as you'd do in a C# class. Instead, we stored these methods/functions
in the global context, and referenced them in Car and SuperCar, to avoid the
memory leaks that were discussed earlier in this chapter. You can, however,
defi ne Drive() inside Car, and Fly() inside SuperCar, without losing any
functionality.
If you comment the execution of this.inheritsFrom(name) from SuperCar, it won't
inherit the capabilities of Car any more. If you make this test in FireFox,
you'll see the following eloquent error message in the Error Console window of
Firefox:
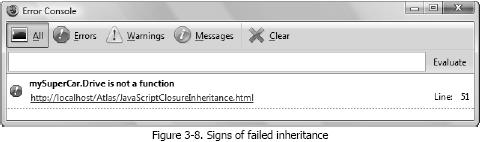
The problem with the presented inheritance solution is that it's not very
elegant. Writing all functions and classes in the global context can quickly
degenerate into chaos; and things get even more complicated if you want to have
classes that have functions with the same name. Needless to say, this isn't
something you need to be dealing with when writing your code. Luckily,
JavaScript has a very neat feature that allows us implement inheritance in a
much cleaner way: prototyping.
Inheritance Using Prototyping
Once again, prototyping can help us implement an OOP feature in a more elegant
way than when using closures. Prototype-based inheritance makes use of the
behavior of JavaScript prototypes. When accessing a member of a function, that
member will be looked for in the function itself. If it's not found there, the
member is looked for in the function's prototype. If it's still not found, the
member is looked for in the prototype's prototype, and so on until the
prototype of the implicit Object object.
In closure-based inheritance, the derived class inherits the base class methods
and properties by "loading" them into itself. Here's the code again for your
reference:
// class SuperCar
function SuperCar(name)
{
// implement closure inheritance
this.inheritsFrom = Car;
this.inheritsFrom(name);
// SuperCar knows how to fly
this.Fly = Fly;
}
When implementing inheritance through prototyping, we can "load" the base class
properties and methods by adding them to the derived class prototype. That way,
an object of the derived class will have access to the class methods and
properties, but also to the base class methods and properties since they exist
in the derived class prototype. To successfully implement prototype-based
inheritance with JavaScript, you need to:
-
Add a base class instance to the derived class prototype property, as in
SuperCar.prototype = new Car(). This creates Car as SuperCar's prototype.
-
The prototype property has a constructor property that needs to point back to
the function itself. Since now the SuperCar's prototype is a Car, its
constructor property points back to the constructor of Car. To fi x this, we
need to set the constructor property of the prototype property of the derived
class to the class itself, as in SuperCar.prototype.constructor = SuperCar.
-
Create the derived class constructor, and call the base class constructor from
there, eventually passing any necessary parameters. In other words, when a new
SuperCar is instantiated, its base class constructor should also execute, to
ensure correct base class functionality.
-
Add any additional derived class members or functions to its prototype.
This is so very complicated! In practice you'll fi nd that the code doesn't look
that scary, although the complete theory is a little more complex than this. A
nice article describing a few additional theoretical aspects can be found at
http://mckoss.com/ jscript/object.htm.
The new implementation of Car and SuperCar, this time using prototypes, is the
following, with the inheritance mechanism highlighted. The Drive() and Fly()
methods have also been created through prototyping, although the old version
using closures would work as well. The code can be checked online at
http://www.cristiandarie.ro/seo-asp/JavaScriptPrototypeInheritance.html.
<script type="text/javascript">
// class Car
function Car(name)
{
// create the Name property
this.Name = name;
}
// Car.Drive() method
Car.prototype.Drive = function()
{
document.write("My name is " + this.Name + " and I'm driving. <br />");
}
// SuperCar inherits from Car
SuperCar.prototype = new Car();
SuperCar.prototype.constructor = SuperCar;
// class SuperCar
function SuperCar(name)
{
// call base class constructor
Car.call(this, name);
}
// SuperCar.Fly() method
SuperCar.prototype.Fly = function()
{
document.write("My name is " + this.Name + " and I'm flying! <br />");
}
// create a new Car and then Drive
var myCar = new Car("Car");
myCar.Drive();
// create SuperCar object
var mySuperCar = new SuperCar("SuperCar");
// SuperCar knows how to drive
mySuperCar.Drive();
// SuperCar knows how to fly
mySuperCar.Fly();
</script>
Here, instead of creating a Car instance in SuperCar's constructor, we declare
Car as SuperCar's prototype.
Related Links
Test your Oops knowledge with
our multiple choice questions!
Object Oriented Programming Interview Questions
What
is object oriented programming (OOP)?
The object oriented programming is commonly known as OOP. Most of the languages
are developed using OOP concept. Object-oriented programming (OOP) is a
programming concept that uses "objects" to develop a system.........
What
are the various elements of OOP?
Various elements of OOP are.........
Explain
an object, class and Method.
An object is an entity that keeps together state and behaviors.
For instance, a car encapsulates state such as red color, 900 cc etc and
behaviors as 'Start', 'Stop' etc., so does an object...............
Define
Encapsulation and Information Hiding in OOP.
Encapsulation means keeping actions and attributes together under a single unit.
This can also be understood using a motor bike example. A bike has actions such
as 'switch on light', 'horn' etc. and attributes such specific color, size,
weight etc..............
Explain Inheritance
and Polymorphism in OOP.
Inheritance concept in OOP allows us to create a new class using an existing
one. It also allows the new class to add its own functionality............
|